Organizing Large CSS Files with Scalable Architecture | Web Formatter Blog
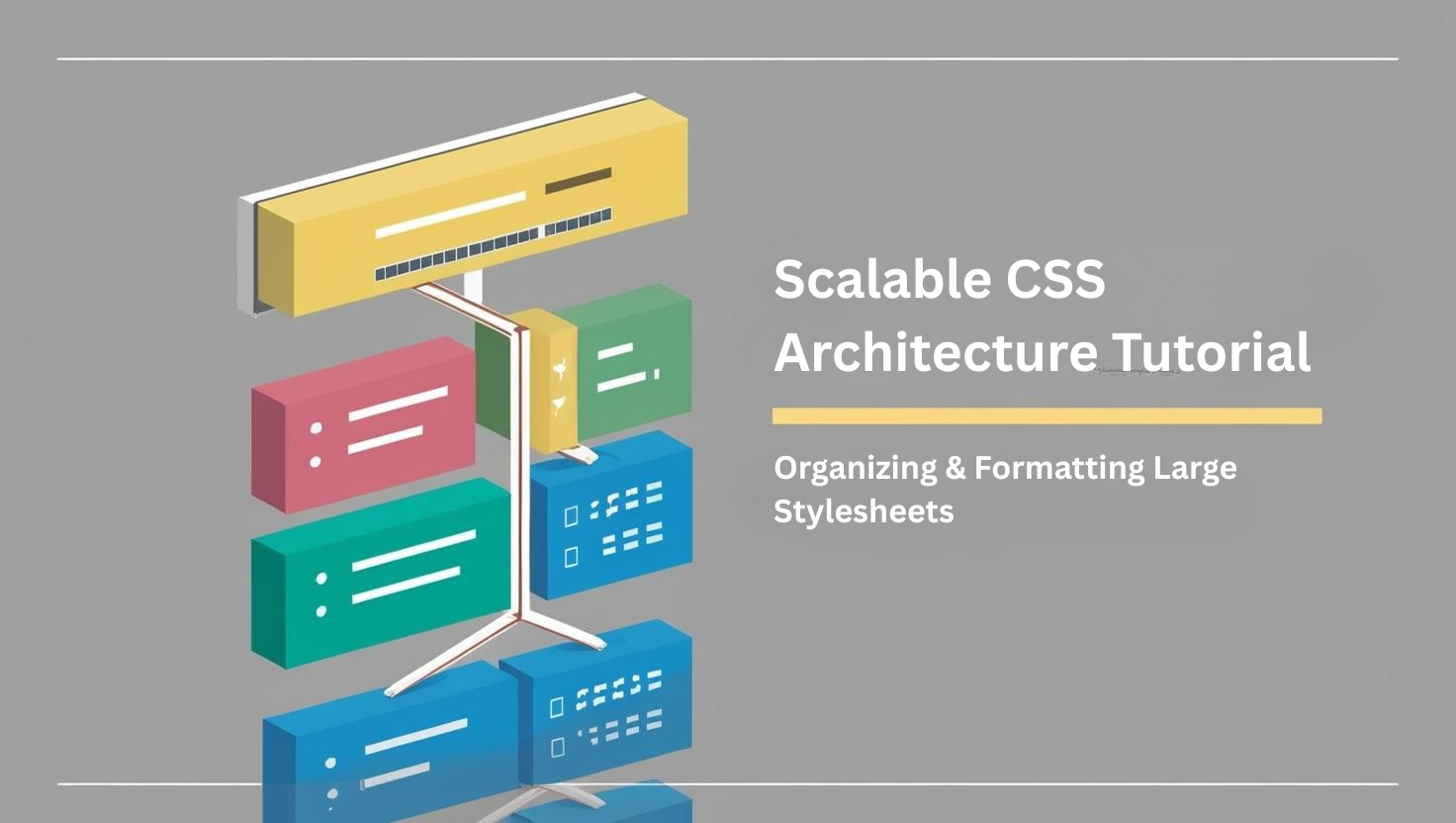
Organizing Large CSS Files with Scalable Architecture
Introduction
As web projects grow in size and complexity, managing CSS becomes increasingly challenging. What starts as a simple stylesheet can quickly evolve into thousands of lines of tangled code, leading to specificity wars, redundant declarations, and maintenance nightmares.
This guide explores how to structure and format CSS for large-scale projects with maintainability in mind. We'll cover various CSS architecture methodologies, file organization strategies, formatting conventions, and tools that can help you keep your styles organized and scalable.
CSS Architecture Principles
Before diving into specific methodologies, let's establish some core principles that any good CSS architecture should follow:
- Predictability: CSS should behave as expected. Developers should be able to make changes without fear of unintended consequences.
- Reusability: Components should be designed for reuse to minimize code duplication.
- Maintainability: Code should be easy to update and extend as requirements change.
- Scalability: The architecture should work just as well for 10,000 lines of CSS as it does for 100.
- Clarity: Naming conventions and organization should make it clear what each piece of CSS does and where it applies.
CSS Methodology Comparison
Several methodologies have emerged to address the challenges of CSS at scale. Let's compare the most popular approaches:
BEM (Block Element Modifier)
BEM is a naming convention that divides components into Blocks, Elements, and Modifiers. This creates a clear relationship between HTML and CSS and helps avoid specificity issues.
.card {} /* Block */
.card__title {} /* Element */
.card__image {} /* Element */
.card--featured {} /* Modifier */
Pros:
- Clear component relationships
- Reduces specificity issues
- Self-documenting class names
Cons:
- Long class names
- Can be verbose in HTML
- Doesn't address file organization
SMACSS (Scalable and Modular Architecture for CSS)
SMACSS categorizes CSS rules into five types: Base, Layout, Module, State, and Theme. This categorization helps organize styles based on their purpose and scope.
/* Base rules */
body, p, h1, h2, h3 { margin: 0; padding: 0; }
/* Layout rules */
.l-header { height: 80px; }
.l-sidebar { width: 25%; }
/* Module rules */
.btn { display: inline-block; }
.btn-primary { background: blue; }
/* State rules */
.is-active { font-weight: bold; }
.is-hidden { display: none; }
/* Theme rules (optional) */
.theme-dark .btn { background: #333; }
Pros:
- Comprehensive approach to organization
- Separates concerns effectively
- Provides guidelines for file structure
Cons:
- More complex to learn and implement
- Some categories can overlap
- Requires team discipline
OOCSS (Object-Oriented CSS)
OOCSS focuses on separating structure from skin and container from content. This promotes reusability and reduces redundancy.
Pros:
- Highly reusable components
- Reduces CSS file size
- Encourages composition
Cons:
- Can lead to "class soup" in HTML
- May be harder to understand component relationships
- Requires careful planning
Atomic CSS
Atomic CSS (or Functional CSS) uses small, single-purpose classes that each do one thing. Frameworks like Tailwind CSS follow this approach.
John Doe
Frontend Developer
Pros:
- Eliminates the need to write custom CSS
- Consistent design constraints
- Reduces CSS file size (with proper tooling)
Cons:
- HTML can become verbose
- Learning curve for utility names
- Can be harder to make global changes
Methodology Comparison Table
Methodology | Focus | Learning Curve | Best For |
---|---|---|---|
BEM | Naming convention | Low | Component-based projects |
SMACSS | Categorization | Medium | Large, complex applications |
OOCSS | Separation of concerns | Medium | Design systems with many shared styles |
Atomic CSS | Single-purpose classes | High | Rapid prototyping, design consistency |
File Organization
How you organize your CSS files is just as important as the methodology you choose. Here are some common approaches:
By Type (SMACSS-inspired)
styles/
├── base/
│ ├── reset.css
│ ├── typography.css
│ └── variables.css
├── layout/
│ ├── grid.css
│ ├── header.css
│ └── footer.css
├── modules/
│ ├── buttons.css
│ ├── cards.css
│ └── forms.css
├── states/
│ └── states.css
└── main.css
By Component (BEM-inspired)
styles/
├── settings/
│ ├── variables.css
│ └── mixins.css
├── elements/
│ ├── typography.css
│ └── forms.css
├── components/
│ ├── button.css
│ ├── card.css
│ ├── navigation.css
│ └── modal.css
├── utilities/
│ └── helpers.css
└── main.css
By Feature (for larger applications)
src/
├── features/
│ ├── authentication/
│ │ ├── components/
│ │ │ ├── login-form.css
│ │ │ └── signup-form.css
│ │ └── pages/
│ │ ├── login-page.css
│ │ └── signup-page.css
│ ├── dashboard/
│ │ ├── components/
│ │ │ ├── widget.css
│ │ │ └── chart.css
│ │ └── pages/
│ │ └── dashboard.css
├── shared/
│ ├── components/
│ │ ├── button.css
│ │ └── modal.css
│ └── styles/
│ ├── variables.css
│ └── global.css
└── main.css
Regardless of the approach you choose, consider these best practices:
- Keep files small and focused on a single responsibility
- Use a consistent naming convention for files
- Create an index file that imports everything in the correct order
- Consider using a build tool to bundle CSS files
Formatting Rules
Consistent formatting makes CSS easier to read and maintain. Here are some recommended formatting rules:
Property Order
Group related properties together. One common approach is:
- Positioning (position, top, right, z-index)
- Box model (display, width, height, margin, padding)
- Typography (font, line-height, text-align)
- Visual (color, background, border, box-shadow)
- Misc (cursor, overflow, transition)
.element {
/* Positioning */
position: absolute;
top: 0;
right: 0;
z-index: 10;
/* Box Model */
display: flex;
width: 100%;
padding: 1rem;
/* Typography */
font-family: sans-serif;
font-size: 1rem;
text-align: center;
/* Visual */
color: #333;
background-color: #fff;
border: 1px solid #ddd;
border-radius: 4px;
/* Misc */
cursor: pointer;
transition: all 0.3s ease;
}
Selector Format
- One selector per line in multi-selector rulesets
- Space after the selector and before the opening brace
- Each property on its own line
- Space after the colon in properties
- Semicolon after each property, including the last one
- Closing brace on a new line
.selector-1,
.selector-2,
.selector-3 {
property: value;
property: value;
}
/* Not */
.selector-1, .selector-2, .selector-3 {
property:value;
property:value
}
Comments
Use comments to explain the purpose of code sections and any non-obvious decisions.
/* ==========================================================================
Section comment block
========================================================================== */
/* Sub-section comment block
========================================================================== */
/**
* Short description using Doxygen-style comment format
*
* Long description first sentence starts here and continues on this line.
* Further paragraphs come after blank lines.
*/
/* Basic comment */
Using Preprocessors
CSS preprocessors like Sass, Less, and Stylus can enhance your CSS architecture with features like variables, nesting, mixins, and imports. Here's how to use them effectively:
Variables for Design Tokens
// _variables.scss
$color-primary: #3b82f6;
$color-secondary: #10b981;
$color-text: #1f2937;
$color-background: #ffffff;
$font-family-base: 'Inter', sans-serif;
$font-size-base: 1rem;
$line-height-base: 1.5;
$spacing-unit: 0.25rem;
$border-radius: 0.25rem;
// Usage
.button {
background-color: $color-primary;
color: $color-background;
font-family: $font-family-base;
padding: $spacing-unit * 4;
border-radius: $border-radius;
}
Mixins for Reusable Patterns
// _mixins.scss
@mixin flex-center {
display: flex;
align-items: center;
justify-content: center;
}
@mixin truncate {
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
}
@mixin responsive($breakpoint) {
@if $breakpoint == sm {
@media (min-width: 640px) { @content; }
} @else if $breakpoint == md {
@media (min-width: 768px) { @content; }
} @else if $breakpoint == lg {
@media (min-width: 1024px) { @content; }
} @else if $breakpoint == xl {
@media (min-width: 1280px) { @content; }
}
}
// Usage
.card {
@include flex-center;
.card__title {
@include truncate;
}
@include responsive(md) {
flex-direction: row;
}
}
Nesting (Use Sparingly)
While nesting can make your code more readable, excessive nesting can lead to specificity issues and overly complex selectors. Limit nesting to 3 levels or less.
File Structure with Partials
scss/
├── abstracts/
│ ├── _variables.scss
│ ├── _functions.scss
│ ├── _mixins.scss
│ └── _placeholders.scss
├── base/
│ ├── _reset.scss
│ ├── _typography.scss
│ └── _animations.scss
├── components/
│ ├── _buttons.scss
│ ├── _carousel.scss
│ ├── _forms.scss
│ └── _modal.scss
├── layout/
│ ├── _header.scss
│ ├── _footer.scss
│ ├── _grid.scss
│ └── _navigation.scss
├── pages/
│ ├── _home.scss
│ └── _contact.scss
├── themes/
│ ├── _light.scss
│ └── _dark.scss
└── main.scss
CSS Modules and CSS-in-JS
Modern frontend frameworks often use CSS Modules or CSS-in-JS solutions to scope styles to components and avoid global namespace conflicts.
CSS Modules
CSS Modules automatically scope class names to components, preventing style leakage.
CSS-in-JS
CSS-in-JS libraries like styled-components or Emotion allow you to write CSS directly in your JavaScript files.
// Button.jsx with styled-components
import React from 'react';
import styled from 'styled-components';
const ButtonBase = styled.button`
padding: 0.5rem 1rem;
border-radius: 4px;
font-weight: 500;
border: none;
cursor: pointer;
transition: background-color 0.2s;
`;
const PrimaryButton = styled(ButtonBase)`
background-color: #3b82f6;
color: white;
&:hover {
background-color: #2563eb;
}
`;
const SecondaryButton = styled(ButtonBase)`
background-color: #e5e7eb;
color: #1f2937;
&:hover {
background-color: #d1d5db;
}
`;
function Button({ children, variant = 'primary', ...props }) {
const ButtonComponent = variant === 'primary' ? PrimaryButton : SecondaryButton;
return {children} ;
}
export default Button;
Linting and Formatting Tools
Automated tools can help enforce your CSS architecture and formatting rules:
Stylelint
Stylelint is a powerful linter that can enforce conventions, catch errors, and sort properties.
// .stylelintrc.json
{
"extends": "stylelint-config-standard",
"plugins": [
"stylelint-order"
],
"rules": {
"color-hex-case": "lower",
"color-hex-length": "short",
"max-nesting-depth": 3,
"selector-class-pattern": "^[a-z][a-zA-Z0-9]*$|^[a-z][a-zA-Z0-9]*(__[a-z][a-zA-Z0-9]*)?$|^[a-z][a-zA-Z0-9]*(__[a-z][a-zA-Z0-9]*)?--[a-z][a-zA-Z0-9]*$",
"order/properties-alphabetical-order": true
}
}
Prettier
Prettier can automatically format your CSS files to ensure consistent styling.
// .prettierrc
{
"singleQuote": true,
"printWidth": 100,
"tabWidth": 2
}
CSS Comb
CSS Comb can sort properties according to a predefined order.
// .csscomb.json
{
"remove-empty-rulesets": true,
"always-semicolon": true,
"color-case": "lower",
"block-indent": " ",
"color-shorthand": true,
"element-case": "lower",
"eof-newline": true,
"leading-zero": false,
"quotes": "double",
"sort-order-fallback": "abc",
"space-before-colon": "",
"space-after-colon": " ",
"space-before-combinator": " ",
"space-after-combinator": " ",
"space-between-declarations": "\\n",
"space-before-opening-brace": " ",
"space-after-opening-brace": "\\n",
"space-after-selector-delimiter": "\\n",
"space-before-selector-delimiter": "", "\\n",
"space-after-selector-delimiter": "\\n",
"space-before-selector-delimiter": "",
"space-before-closing-brace": "\\n",
"sort-order": [
"position",
"top",
"right",
"bottom",
"left",
"z-index",
"display",
"flex-direction",
"flex-wrap",
"justify-content",
"align-items",
"width",
"height",
"margin",
"padding",
"font",
"color",
"background",
"border",
"transition"
]
}
Case Study: Refactoring Legacy CSS
Let's look at a practical example of refactoring legacy CSS into a more maintainable architecture.
The refactoring process typically involves these steps:
- Audit the existing CSS to identify patterns and components
- Choose an appropriate methodology (BEM, SMACSS, etc.)
- Create a new file structure
- Refactor one component at a time
- Update HTML to use the new class names
- Test thoroughly to ensure nothing breaks
- Document the new architecture for the team
Conclusion
A well-structured CSS architecture is essential for maintaining large-scale web projects. By adopting a consistent methodology, organizing your files logically, following formatting conventions, and using the right tools, you can create CSS that is easier to maintain, scale, and collaborate on.
Remember that there's no one-size-fits-all solution. The best approach depends on your project's size, team structure, and specific requirements. Start with these principles and adapt them to your needs.
For your next project, consider implementing these practices from the beginning. For existing projects, start with small, incremental improvements rather than attempting a complete rewrite all at once.