Organizing Large CSS Files with Scalable Architecture | Web Formatter Blog
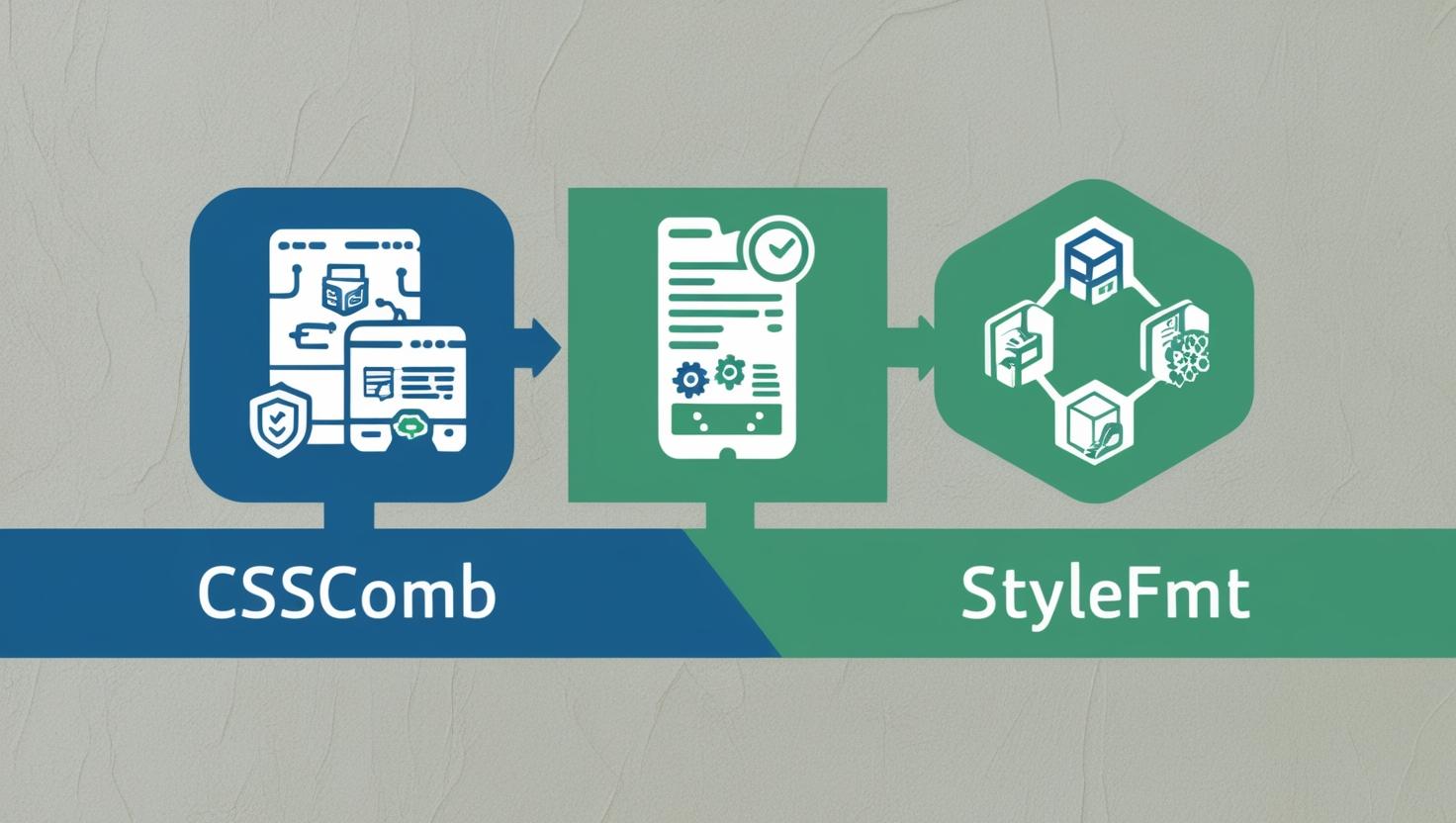
Organizing Large CSS Files with Scalable Architecture
A comprehensive guide to structuring and formatting CSS for maintainable large-scale projects.
Introduction to CSS Architecture
As web projects grow in size and complexity, managing CSS becomes increasingly challenging. CSS architecture refers to the organization, structure, and methodology used to write and maintain CSS code. A well-planned CSS architecture helps teams work more efficiently, reduces bugs, and makes codebases more maintainable over time.
This guide explores various approaches to CSS architecture, formatting conventions, and tools that can help you manage large-scale CSS projects effectively.
Challenges of Large CSS Codebases
Before diving into solutions, it's important to understand the common challenges that arise with large CSS codebases:
- Specificity conflicts: Overlapping selectors causing unexpected styling outcomes
- Cascade complexity: Difficulty tracking how styles cascade and override each other
- Naming collisions: Class names that unintentionally conflict across components
- Code duplication: Repeated CSS patterns leading to bloated stylesheets
- Poor maintainability: Difficulty updating styles without breaking existing features
- Lack of scalability: Architecture that doesn't accommodate project growth
- Team coordination issues: Multiple developers working on the same stylesheets
A good CSS architecture addresses these challenges through organization, conventions, and tooling.
CSS Architecture Methodologies
Several established methodologies provide frameworks for organizing CSS. Each has its strengths and is suited to different types of projects.
BEM (Block Element Modifier)
BEM is a naming convention that creates a clear relationship between HTML and CSS. It divides components into blocks, elements, and modifiers.
/* Block component */
.card {
background: white;
border-radius: 4px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
/* Element */
.card__title {
font-size: 1.25rem;
font-weight: bold;
}
/* Modifier */
.card--featured {
border: 2px solid #3498db;
}
BEM's main advantages include:
- Clear, explicit naming that shows relationships between components
- Reduced specificity issues by avoiding nested selectors
- Self-documenting class names that indicate purpose
- Modular approach that works well with component-based architectures
SMACSS (Scalable and Modular Architecture for CSS)
SMACSS categorizes CSS rules into five types: Base, Layout, Module, State, and Theme. This categorization helps organize styles based on their purpose.
/* Base rules */
body, p, h1, h2, h3 {
margin: 0;
padding: 0;
}
/* Layout rules */
.l-header {
height: 80px;
}
/* Module rules */
.btn {
display: inline-block;
padding: 0.5rem 1rem;
}
/* State rules */
.is-active {
font-weight: bold;
}
SMACSS is particularly useful for larger projects where clear categorization helps manage complexity.
OOCSS (Object-Oriented CSS)
OOCSS focuses on separating structure from skin and container from content. This promotes reusability and reduces code duplication.
/* Structure */
.btn {
display: inline-block;
padding: 0.5rem 1rem;
border-radius: 4px;
}
/* Skin */
.btn-primary {
background-color: #3498db;
color: white;
}
.btn-secondary {
background-color: #95a5a6;
color: white;
}
OOCSS principles help create more reusable components and reduce stylesheet size.
Atomic CSS
Atomic CSS (or Functional CSS) uses small, single-purpose classes that each do one thing. This approach prioritizes reusability at the class level.
/* Atomic classes */
.flex { display: flex; }
.p-2 { padding: 0.5rem; }
.m-2 { margin: 0.5rem; }
.text-center { text-align: center; }
.bg-white { background-color: white; }
.text-black { color: black; }
Frameworks like Tailwind CSS have popularized this approach, which reduces CSS file size but increases HTML class attributes.
File Organization Strategies
How you organize your CSS files is as important as the methodology you choose. Here are common approaches to file organization:
Component-Based Organization
In this approach, CSS is organized by UI components, with each component having its own CSS file.
styles/
├── components/
│ ├── button.css
│ ├── card.css
│ └── form.css
├── base/
│ ├── reset.css
│ └── typography.css
└── main.css
This organization works well with component-based frameworks like React, Vue, or Angular.
Function-Based Organization
Function-based organization groups CSS by its purpose or function rather than by component.
styles/
├── base/
│ ├── reset.css
│ └── typography.css
├── layout/
│ ├── grid.css
│ └── header.css
├── components/
│ ├── button.css
│ └── card.css
└── main.css
This approach aligns well with the SMACSS methodology and works for projects where components share many styles.
Hybrid Approaches
Many projects benefit from a hybrid approach that combines aspects of both component-based and function-based organization.
styles/
├── base/
│ ├── reset.css
│ └── typography.css
├── components/
│ ├── button.css
│ └── card.css
├── layout/
│ ├── grid.css
│ └── header.css
└── main.css
This organization provides flexibility while maintaining clear structure.
Naming Conventions
Consistent naming conventions make CSS more predictable and easier to maintain. Beyond BEM, here are some general naming principles:
-
Use meaningful names:{" "}
.primary-button
is better than.btn1
- Be consistent with formatting: Choose kebab-case, camelCase, or another style and stick with it
-
Use namespaces: Prefixes like
.l-
{" "} for layout,.c-
for components -
Indicate state: Use prefixes like{" "}
.is-
or.has-
for state classes -
Avoid overly specific names:{" "}
.card-title
is better than{" "}.homepage-featured-article-card-title
Example of a naming convention system:
/* Component namespace */
.c-card { /* Component styles */ }
.c-button { /* Component styles */ }
/* Layout namespace */
.l-header { /* Layout styles */ }
.l-footer { /* Layout styles */ }
/* Utility namespace */
.u-mt-1 { margin-top: 1rem; }
.u-mb-1 { margin-bottom: 1rem; }
/* State namespace */
.is-active { /* State styles */ }
.is-hidden { /* State styles */ }
CSS Formatting Rules
Consistent formatting makes CSS more readable and easier to maintain. Here are some best practices:
Indentation and Spacing
Consistent indentation and spacing improve readability:
Property Ordering
Consistent property ordering makes it easier to scan and find specific properties:
- Alphabetical: Simple and easy to remember
- Type-based: Grouped by positioning, display, colors, text, etc.
- Concentric: Outside-in (position, margin, border, padding, content)
Example of type-based ordering:
.element {
/* Positioning */
position: absolute;
top: 0;
right: 0;
z-index: 10;
/* Box Model */
display: flex;
width: 100%;
padding: 1rem;
/* Typography */
font-family: sans-serif;
font-size: 1rem;
text-align: center;
/* Visual */
color: #333;
background-color: #fff;
border: 1px solid #ddd;
border-radius: 4px;
/* Misc */
cursor: pointer;
transition: all 0.3s ease;
}
Comments and Documentation
Well-placed comments help explain complex CSS and document architectural decisions:
/* ==========================================================================
Section comment block
========================================================================== */
/* Sub-section comment block
========================================================================== */
/**
* Short description using Doxygen-style comment format
*
* Long description first sentence starts here and continues on this line.
* Further paragraphs come after blank lines.
*/
/* Basic comment */
Modern CSS Approaches
Modern web development has introduced new approaches to CSS that help address scalability challenges.
CSS Modules
CSS Modules scope CSS by automatically creating unique class names during the build process.
/* Button.module.css */
.button {
padding: 8px 16px;
border-radius: 4px;
background-color: #3498db;
color: white;
border: none;
cursor: pointer;
}
.button:hover {
background-color: #2980b9;
}
// Button.jsx
import styles from './Button.module.css';
function Button({ children, onClick }) {
return (
);
}
export default Button;
CSS Modules eliminate naming collisions and make components more self-contained.
CSS-in-JS
CSS-in-JS libraries allow you to write CSS directly in your JavaScript components.
// Using styled-components
import styled from 'styled-components';
const Button = styled.button`
padding: 8px 16px;
border-radius: 4px;
background-color: #3498db;
color: white;
border: none;
cursor: pointer;
&:hover {
background-color: #2980b9;
}
`;
function App() {
return (
);
}
CSS-in-JS offers benefits like dynamic styling, scoped styles, and co-location of styles with components.
Utility-First CSS
Utility-first CSS frameworks like Tailwind CSS provide low-level utility classes to build designs directly in your markup.
Utility-first CSS reduces the need to write custom CSS and promotes consistency through predefined constraints.
Tools for CSS Architecture
Various tools can help implement and maintain a solid CSS architecture.
CSS Preprocessors
Preprocessors like Sass, Less, and Stylus enhance CSS with variables, mixins, nesting, and more.
// Variables
$primary-color: #3498db;
$border-radius: 4px;
// Mixins
@mixin flex-center {
display: flex;
align-items: center;
justify-content: center;
}
// Nesting
.card {
background: white;
border-radius: $border-radius;
&__title {
font-size: 1.25rem;
font-weight: bold;
}
&__content {
padding: 1rem;
}
&--featured {
border: 2px solid $primary-color;
}
}
Preprocessors facilitate more maintainable and organized CSS through features like partials, imports, and functions.
CSS Linters and Formatters
Tools like Stylelint and Prettier help enforce consistent formatting and catch potential issues.
// .stylelintrc
{
"extends": "stylelint-config-standard",
"plugins": [
"stylelint-order"
],
"rules": {
"color-hex-case": "lower",
"color-hex-length": "short",
"max-nesting-depth": 3,
"selector-class-pattern": "^[a-z][a-zA-Z0-9]*$|^[a-z][a-zA-Z0-9]*(__[a-z][a-zA-Z0-9]*)?$|^[a-z][a-zA-Z0-9]*(__[a-z][a-zA-Z0-9]*)?--[a-z][a-zA-Z0-9]*$",
"order/properties-alphabetical-order": true
}
}
Automated linting and formatting ensure consistency across large teams and codebases.
Build Tools
Modern build tools help optimize CSS for production:
- PostCSS: Transform CSS with JavaScript plugins
- PurgeCSS: Remove unused CSS
- CSS Modules: Scope CSS to components
- CSS Nano: Minify and optimize CSS
- Autoprefixer: Add vendor prefixes automatically
// postcss.config.js
module.exports = {
plugins: [
require('autoprefixer'),
require('postcss-nested'),
require('postcss-custom-properties'),
require('postcss-custom-media'),
require('postcss-custom-selectors'),
require('postcss-flexbugs-fixes'),
require('postcss-preset-env')({
stage: 3,
features: {
'custom-properties': false
}
})
]
}
Refactoring Existing CSS
Refactoring a large, legacy CSS codebase requires a strategic approach:
- Audit the existing CSS to identify patterns and components
- Choose an appropriate methodology (BEM, SMACSS, etc.)
- Create a new file structure
- Refactor one component at a time
- Update HTML to use the new class names
- Test thoroughly to ensure nothing breaks
- Document the new architecture for the team
Conclusion
A well-structured CSS architecture is essential for maintaining large-scale web projects. By adopting a consistent methodology, organizing your files logically, following formatting conventions, and using the right tools, you can create CSS that is easier to maintain, scale, and collaborate on.
Remember that there's no one-size-fits-all solution. The best approach depends on your project's size, team structure, and specific requirements. Start with these principles and adapt them to your needs.
For your next project, consider implementing these practices from the beginning. For existing projects, start with small, incremental improvements rather than attempting a complete rewrite all at once.