Go Formatter CLI + Editor Integration | Web Formatter Blog
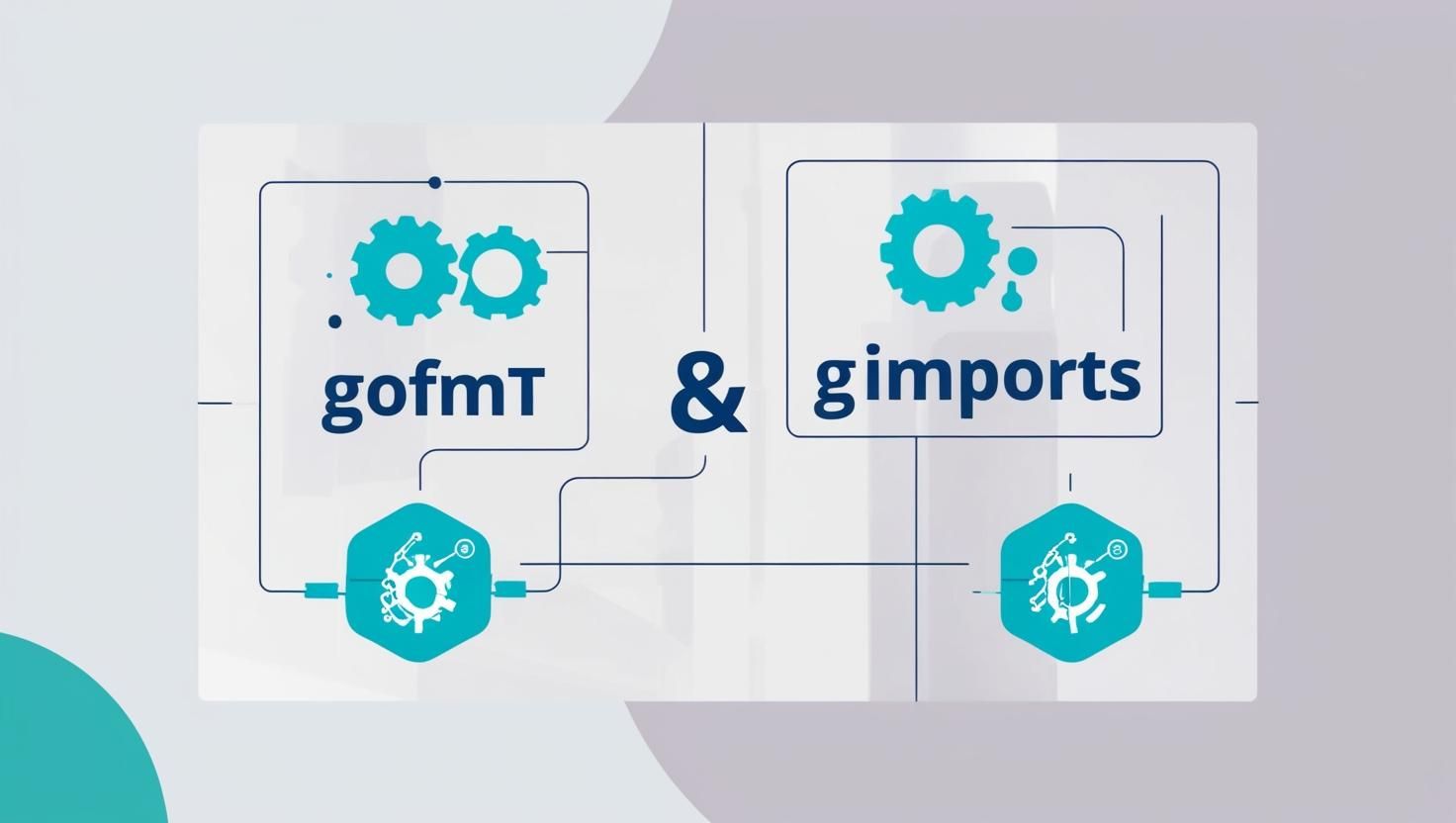
Go Formatter CLI + Editor Integration
A comprehensive guide to formatting Go code with gofmt and goimports.
Introduction to Go Formatting
One of Go's most distinctive features is its approach to code formatting. Unlike many other programming languages where formatting is largely a matter of personal or team preference, Go takes a more opinionated stance. The Go ecosystem provides official tools that automatically format your code according to a standardized style.
In this comprehensive guide, we'll explore the two primary Go formatting tools—gofmt and goimports—and how to integrate them into your development workflow for consistent, clean, and idiomatic Go code.
Why Format Your Go Code?
The benefits of consistent formatting extend far beyond aesthetics:
- Improved readability: Consistently formatted code is easier to scan and comprehend
- Reduced cognitive load: Developers can focus on logic rather than style discrepancies
- Easier collaboration: Standardized formatting eliminates style debates in code reviews
- Faster onboarding: New team members can quickly adapt to the codebase
- Community consistency: Go code looks similar across different projects and organizations
Go's creator Rob Pike famously said, "Gofmt's style is no one's favorite, yet gofmt is everyone's favorite." This philosophy has led to remarkable consistency across the Go ecosystem, where virtually all Go code follows the same formatting conventions.
Understanding gofmt
gofmt
(pronounced "go format") is the standard
formatting tool for Go code. It's included with the Go
installation and provides automatic formatting according to Go's
official style guidelines. Key features include:
- Standardized indentation (tabs for indentation, spaces for alignment)
- Consistent spacing around operators and punctuation
- Proper line wrapping for long statements
- Organized struct fields and interface methods
- Canonical ordering of imports
The tool is non-configurable by design—there are no style options or preferences to set. This deliberate limitation ensures that all Go code follows the same conventions, eliminating style debates and configuration overhead.
Understanding goimports
goimports
is an extension of gofmt
that
adds automatic management of import statements. In addition to all
the formatting that gofmt
does,{" "}
goimports
:
- Adds missing import statements based on the identifiers used in the code
- Removes unused import statements
- Groups and sorts imports according to standard conventions
- Distinguishes between standard library, third-party, and local imports
For most Go developers, goimports
has become the
preferred tool since it handles both formatting and import
management in a single pass.
Installation
Installing gofmt
gofmt
comes bundled with the Go installation, so if
you have Go installed, you already have gofmt
. You
can verify this by running:
go version
gofmt -h
Installing goimports
goimports
is not included in the standard Go
installation and needs to be installed separately:
go install golang.org/x/tools/cmd/goimports@latest
After installation, verify that it's working:
goimports -h
If you encounter any issues, ensure that your Go bin directory
(typically $GOPATH/bin
or $HOME/go/bin
)
is in your PATH.
Basic Usage
Using gofmt
To format a single file and print the result to standard output:
gofmt file.go
To format a file in-place (modifying the original file):
gofmt -w file.go
To format all Go files in a directory and its subdirectories:
gofmt -w .
To see the differences that would be made without actually changing files:
gofmt -d file.go
Using goimports
goimports
has a similar interface to{" "}
gofmt
:
# Print formatted output to stdout
goimports file.go
# Format file in-place
goimports -w file.go
# Format all Go files in current directory and subdirectories
goimports -w .
# Show differences without changing files
goimports -d file.go
Formatting Options
While Go's formatting tools are intentionally non-configurable in terms of style, they do offer a few options:
# Simplify code (eliminates unnecessary code)
gofmt -s -w file.go
# Format and rewrite to use shorter variable declarations where possible
gofmt -s -w file.go
# Control import grouping in goimports
goimports -local github.com/myorg/myproject -w file.go
The -local
flag for goimports
is
particularly useful for organizing imports into groups: standard
library, third-party packages, and your organization's packages.
Code Examples
Basic Formatting Examples
Notice how gofmt
:
- Adds a space after the opening brace of the function
-
Standardizes spacing around the
:=
operator - Uses tabs for indentation consistently
- Normalizes blank lines
Import Management
goimports
makes several improvements:
- Groups imports into a single import block with parentheses
- Sorts standard library imports alphabetically
- Separates third-party imports with a blank line
- Removes the unused import
Complex Formatting Examples
In this more complex example, gofmt
:
- Properly indents and aligns struct fields
- Formats multi-line function calls with proper indentation
- Normalizes indentation in control structures
- Ensures consistent spacing in the import block
Editor Integration
For the best development experience, integrate Go's formatting tools directly into your editor.
Visual Studio Code
VS Code with the official Go extension provides excellent support for Go formatting:
- Install the{" "} Go extension
- Open VS Code settings (File {">"} Preferences {">"} Settings)
- Search for "Go: Format Tool" and select "goimports" from the dropdown
- Enable "Format On Save" for automatic formatting
Configuration in settings.json:
{
"go.formatTool": "goimports",
"editor.formatOnSave": true,
"go.useLanguageServer": true,
"go.lintTool": "golangci-lint",
"[go]": {
"editor.defaultFormatter": "golang.go",
"editor.codeActionsOnSave": {
"source.organizeImports": true
}
}
}
GoLand
JetBrains GoLand includes built-in support for Go formatting:
- Go to Settings/Preferences {">"} Tools {">"} File Watchers
- Click the + button and select "go fmt" or "goimports" from the list
- Configure the scope and options as needed
- Enable "Format on save" in Settings {">"} Editor {">"} Code Style {">"} Go
GoLand also supports running goimports on save:
- Go to Settings {">"} Tools {">"} Actions on Save
- Enable "Reformat code" and "Optimize imports"
Vim and Neovim
For Vim/Neovim, you can use plugins like vim-go or integrate with LSP:
" Using vim-go
Plug 'fatih/vim-go', { 'do': ':GoUpdateBinaries' }
" Configuration
let g:go_fmt_command = "goimports"
let g:go_fmt_autosave = 1
" For Neovim with built-in LSP
lua << EOF
require'lspconfig'.gopls.setup{
on_attach = function(client)
-- Format on save
vim.api.nvim_command[[autocmd BufWritePre lua vim.lsp.buf.formatting_sync()]]
end,
}
EOF
Emacs
For Emacs, you can use go-mode with gofmt integration:
(use-package go-mode
:ensure t
:config
(setq gofmt-command "goimports")
(add-hook 'before-save-hook 'gofmt-before-save))
Or with LSP support:
(use-package lsp-mode
:ensure t
:commands (lsp lsp-deferred)
:hook (go-mode . lsp-deferred)
:config
(setq lsp-gopls-staticcheck t)
(setq lsp-gopls-complete-unimported t))
Automating Formatting
Git Pre-commit Hooks
Ensure code is properly formatted before it's committed using Git hooks:
#!/bin/sh
# .git/hooks/pre-commit
# Make sure this file is executable (chmod +x)
STAGED_GO_FILES=$(git diff --cached --name-only --diff-filter=ACM | grep ".go$")
if [[ "$STAGED_GO_FILES" = "" ]]; then
exit 0
fi
PASS=true
for FILE in $STAGED_GO_FILES
do
# Format the file
goimports -w "$FILE"
# Add the formatted file back to staging
git add "$FILE"
done
exit 0
For a more robust solution, consider using pre-commit framework:
# .pre-commit-config.yaml
repos:
- repo: https://github.com/dnephin/pre-commit-golang
rev: master
hooks:
- id: go-fmt
- id: go-imports
- id: go-vet
- id: go-lint
CI/CD Integration
Add formatting checks to your CI pipeline to catch issues early. Example for GitHub Actions:
name: Go Formatting
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
format:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Go
uses: actions/setup-go@v3
with:
go-version: '1.20'
- name: Install goimports
run: go install golang.org/x/tools/cmd/goimports@latest
- name: Check formatting
run: |
# Check if gofmt would make any changes
gofmt_output=$(gofmt -l .)
if [ -n "$gofmt_output" ]; then
echo "The following files need formatting with gofmt:"
echo "$gofmt_output"
exit 1
fi
# Check if goimports would make any changes
goimports_output=$(goimports -l .)
if [ -n "$goimports_output" ]; then
echo "The following files need formatting with goimports:"
echo "$goimports_output"
exit 1
fi
Best Practices
To get the most out of Go's formatting tools:
- Format on save: Configure your editor to automatically format code when saving files
- Use goimports instead of gofmt for the added benefit of import management
- Add formatting checks to CI to ensure all code in the repository is properly formatted
- Use the -local flag with goimports to properly group your organization's packages
- Don't fight the formatter: Embrace Go's opinionated approach rather than trying to work around it
- Run gofmt -s to simplify code where possible
- Combine with other tools like golint, go vet, and staticcheck for comprehensive code quality checks
Troubleshooting Common Issues
When working with Go's formatting tools, you might encounter these common issues:
Issue | Solution |
---|---|
goimports not found | Ensure Go bin directory is in your PATH or use absolute path |
Imports not organizing as expected |
Use the -local flag to specify your
organization's import prefix
|
Editor not formatting on save | Check editor configuration and ensure the Go extension is properly installed |
Formatting breaking code | This usually indicates a syntax error in your code that needs to be fixed |
Slow performance on large files | Consider using gofumpt for faster formatting or format specific files instead of entire directories |
Conclusion
Go's approach to code formatting is a fundamental aspect of the language's philosophy. By providing standard, non-configurable formatting tools, Go eliminates style debates and ensures consistency across the entire ecosystem.
While it might initially feel restrictive to developers accustomed to configurable formatters, most Go developers quickly appreciate the benefits of standardized formatting. It reduces cognitive load, simplifies collaboration, and ultimately allows everyone to focus on what matters most: writing clear, efficient, and correct code.
By integrating tools like gofmt and goimports into your workflow—whether through editor integration, git hooks, or CI/CD pipelines—you'll ensure your Go code is consistently formatted according to community standards, making it more readable and maintainable for everyone who works with it.
Remember: in Go, formatting isn't just a matter of aesthetics—it's a core part of the language's design philosophy and a key contributor to the readability and maintainability of Go codebases everywhere.