Best Practices for JSON Formatting & Schema Validation | Web Formatter Blog
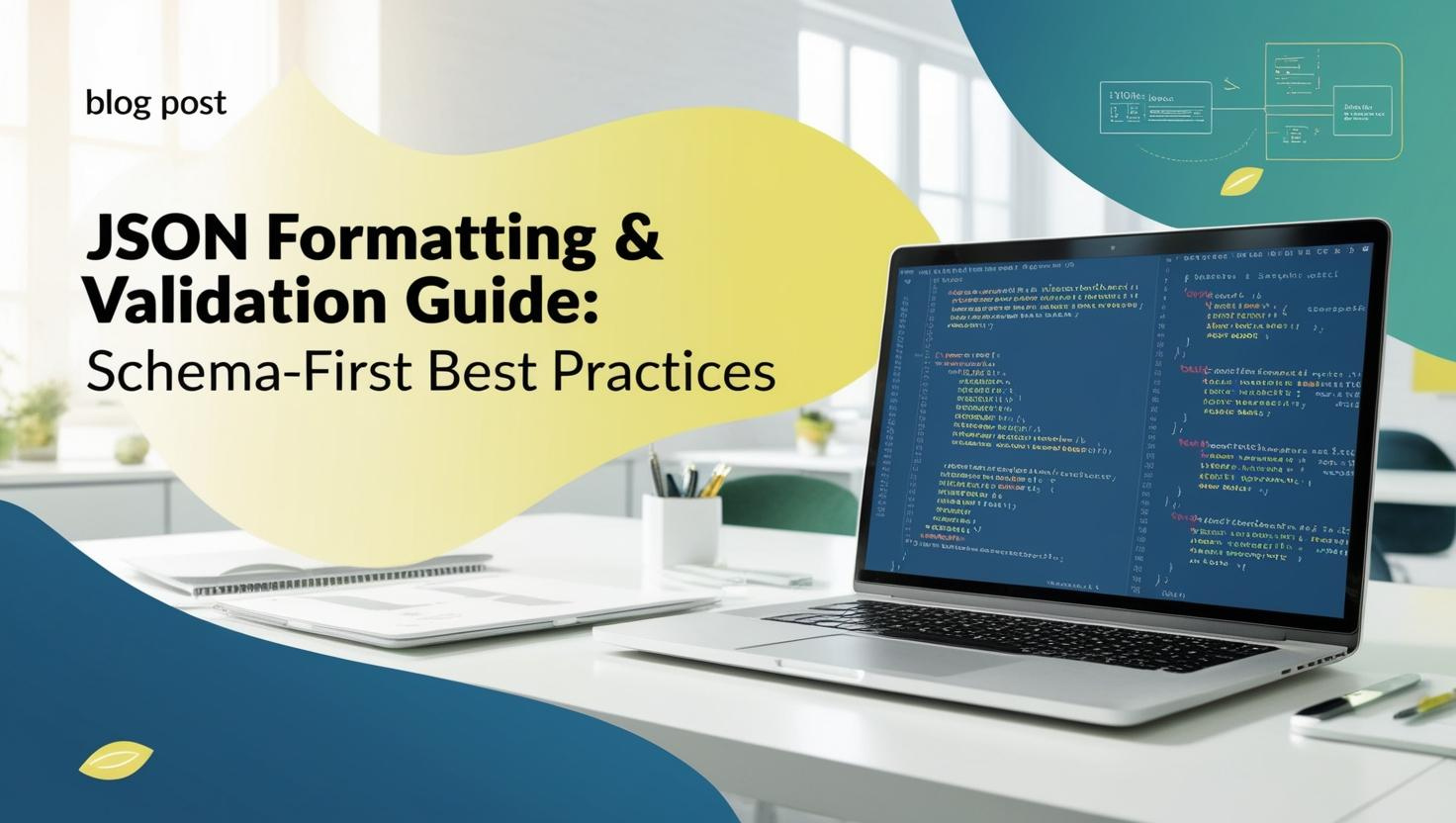
{post.title}
{post.excerpt}
{post.tags.length > 0 && (Web Formatter Team
Code Formatting Experts
Introduction to JSON Formatting
JavaScript Object Notation (JSON) has become the de facto standard for data exchange in web applications. Its simplicity and readability make it ideal for both humans and machines. However, as JSON documents grow in complexity, proper formatting and validation become crucial for maintainability and reliability.
In this guide, we'll explore best practices for formatting JSON and implementing schema validation to ensure data integrity in your applications.
JSON Basics: A Quick Refresher
Before diving into formatting best practices, let's quickly review the basic structure of JSON:
{
"string": "This is a string value",
"number": 42,
"boolean": true,
"null": null,
"array": [1, 2, 3],
"object": {
"nested": "value"
}
}
JSON supports six data types:
- Strings: Text enclosed in double quotes
- Numbers: Integer or floating-point values
- Booleans: true or false
- null: Represents no value
- Arrays: Ordered lists of values
- Objects: Collections of key-value pairs
JSON Formatting Best Practices
Proper formatting makes JSON more readable and easier to maintain. Here are some best practices:
Consistent Indentation
Use consistent indentation (2 or 4 spaces) to show the hierarchical structure:
{
"user": {
"name": "John Doe",
"email": "[email protected]",
"preferences": {
"theme": "dark",
"notifications": true
}
}
}
Logical Property Ordering
Order properties logically to improve readability:
- Group related properties together
- Place identifier properties (id, name) first
- Consider alphabetical ordering for large objects
{
"id": "user-123",
"name": "John Doe",
"email": "[email protected]",
"created_at": "2023-01-15T12:00:00Z",
"updated_at": "2023-08-01T09:30:00Z",
"settings": {
"theme": "dark",
"language": "en-US",
"notifications": true
}
}
Consistent Naming Conventions
Choose a naming convention and stick with it:
- camelCase: Commonly used in JavaScript
- snake_case: Popular in many APIs
- kebab-case: Less common but used in some systems
Avoid mixing conventions within the same document:
// Good: Consistent snake_case
{
"user_id": "123",
"first_name": "John",
"last_name": "Doe",
"email_address": "[email protected]"
}
// Bad: Mixed conventions
{
"userId": "123",
"first_name": "John",
"lastName": "Doe",
"email-address": "[email protected]"
}
Array Formatting
For arrays, choose a formatting style based on length and complexity:
// Short arrays on a single line
{
"tags": ["javascript", "json", "formatting"]
}
// Longer arrays with one item per line
{
"permissions": [
"read:users",
"write:users",
"delete:users",
"read:reports",
"write:reports"
]
}
// Arrays of objects always with one object per line
{
"users": [
{ "id": 1, "name": "Alice" },
{ "id": 2, "name": "Bob" },
{ "id": 3, "name": "Charlie" }
]
}
JSON Schema Validation
JSON Schema is a vocabulary that allows you to annotate and validate JSON documents. It helps ensure that your JSON data adheres to a specific structure and contains valid values.
JSON Schema Basics
A JSON Schema document is itself a JSON document that defines the structure, types, and constraints for another JSON document:
{
"$schema": "https://json-schema.org/draft/2020-12/schema",
"type": "object",
"properties": {
"name": {
"type": "string",
"minLength": 1
},
"age": {
"type": "integer",
"minimum": 0
},
"email": {
"type": "string",
"format": "email"
}
},
"required": ["name", "email"]
}
Benefits of Schema Validation
Implementing JSON Schema validation provides several benefits:
- Data Integrity: Ensures data meets your requirements
- Self-Documentation: Schemas serve as documentation for your data structures
- Early Error Detection: Catches data issues before they cause problems
- API Consistency: Ensures consistent data formats in requests and responses
- Code Generation: Can be used to generate types, forms, and documentation
Implementing Schema Validation
Here's how to implement JSON Schema validation in different environments:
Node.js
Using the Ajv library:
// Install Ajv
// npm install ajv
const Ajv = require("ajv");
const ajv = new Ajv();
// Define your schema
const schema = {
type: "object",
properties: {
name: { type: "string" },
age: { type: "integer", minimum: 0 },
email: { type: "string", format: "email" }
},
required: ["name", "email"]
};
// Compile the schema
const validate = ajv.compile(schema);
// Validate data
const data = {
name: "John Doe",
age: 30,
email: "[email protected]"
};
const valid = validate(data);
if (!valid) {
console.log(validate.errors);
}
Browser
Using the same Ajv library in the browser:
// Include Ajv in your HTML
//
// Create a validator
const ajv = new Ajv();
// Define your schema
const schema = {
type: "object",
properties: {
name: { type: "string" },
email: { type: "string", format: "email" }
},
required: ["name", "email"]
};
// Compile the schema
const validate = ajv.compile(schema);
// Validate form data
document.querySelector('form').addEventListener('submit', (event) => {
const formData = {
name: document.getElementById('name').value,
email: document.getElementById('email').value
};
const valid = validate(formData);
if (!valid) {
event.preventDefault();
displayErrors(validate.errors);
}
});
Advanced Schema Features
JSON Schema offers many advanced features for complex validation:
Conditional Validation
{
"type": "object",
"properties": {
"paymentMethod": {
"type": "string",
"enum": ["credit_card", "bank_transfer", "paypal"]
},
"creditCardNumber": {
"type": "string",
"pattern": "^[0-9]{16}$"
}
},
"required": ["paymentMethod"],
"if": {
"properties": {
"paymentMethod": { "const": "credit_card" }
}
},
"then": {
"required": ["creditCardNumber"]
}
}
Custom Formats
// Define a custom format validator
ajv.addFormat("uuid", /^[0-9a-f]{8}-[0-9a-f]{4}-[0-9a-f]{4}-[0-9a-f]{4}-[0-9a-f]{12}$/i);
// Use it in your schema
const schema = {
type: "object",
properties: {
id: { type: "string", format: "uuid" }
}
};
JSON Formatting and Validation Tools
Several tools can help you format and validate JSON:
Online Tools
- JSONLint: Validates and formats JSON
- JSON Schema Validator: Validates JSON against a schema
- JSON Formatter & Validator: Our own tool at Web Formatter
Editor Plugins
- VS Code: Built-in JSON formatting and schema validation
- WebStorm: Excellent JSON support with schema validation
- Sublime Text: Pretty JSON plugin for formatting
Command Line Tools
# Format JSON with jq
cat data.json | jq '.'
# Validate JSON against a schema with jsonschema
jsonschema -i data.json schema.json
Best Practices Summary
To summarize, here are the key best practices for JSON formatting and validation:
- Use consistent formatting with proper indentation and spacing
- Follow a naming convention consistently throughout your JSON
- Order properties logically to improve readability
- Implement JSON Schema validation to ensure data integrity
- Document your schemas to help others understand your data structures
- Use automated tools to enforce formatting and validation
- Consider performance implications for very large JSON documents
Conclusion
Proper JSON formatting and schema validation are essential practices for maintaining clean, reliable, and self-documenting code. By following these best practices, you'll improve the readability of your JSON data, catch errors early, and ensure consistency across your applications.
Remember that the goal is to make your JSON both machine-readable and human-friendly. Well-formatted JSON with proper validation leads to fewer bugs, easier debugging, and a better developer experience.