PHP PSR-12 Guide: Autoformat with PHP-CS-Fixer | Web Formatter Blog
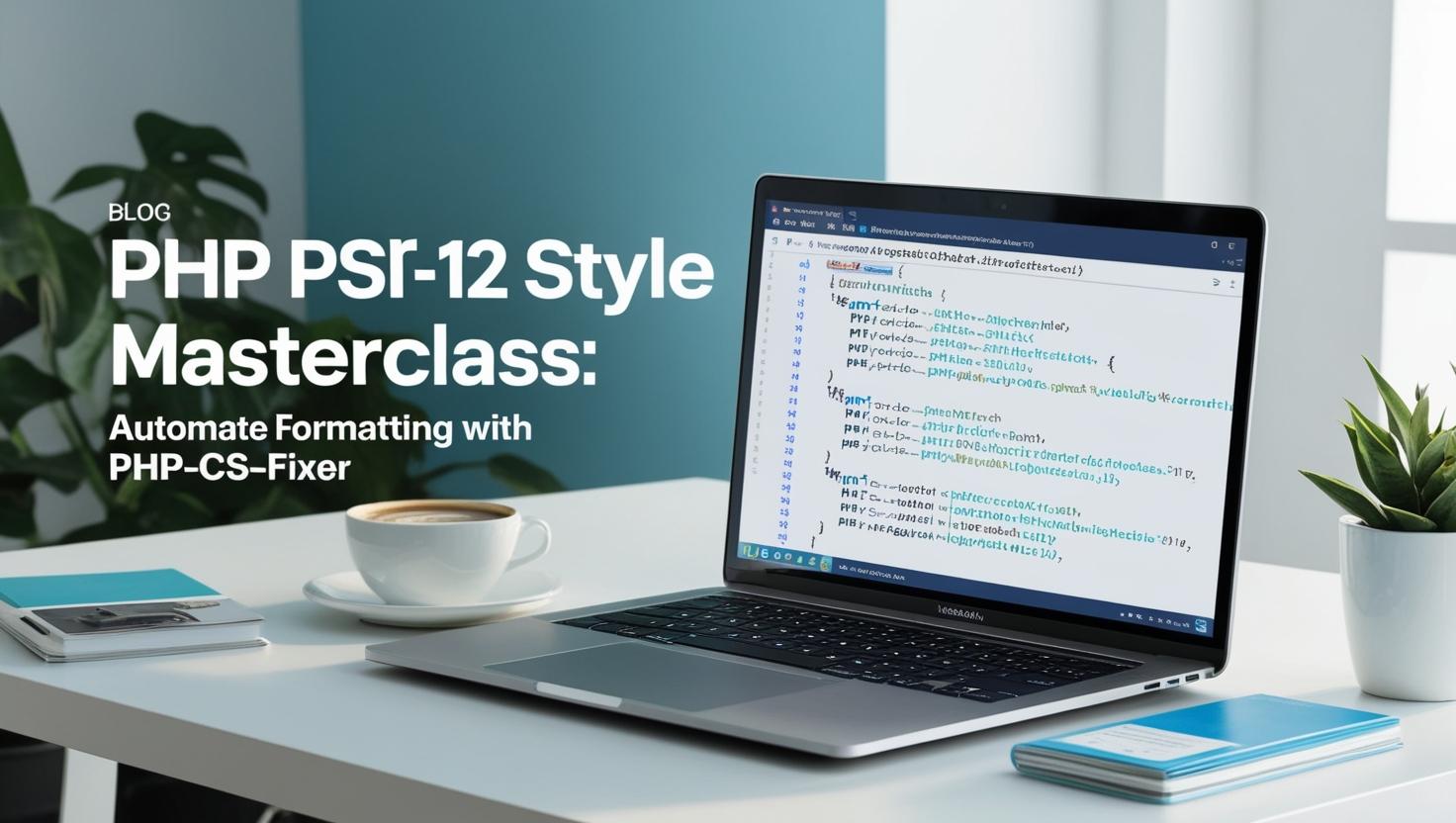
PHP PSR-12 Guide: Autoformat with PHP-CS-Fixer
A comprehensive guide to implementing PSR-12 coding standards in your PHP projects using PHP-CS-Fixer.
Introduction to PSR-12
Consistent code formatting is essential for maintaining readable and maintainable PHP projects. PSR-12 (PHP Standards Recommendation) is the extended coding style guide that builds upon and replaces PSR-2, providing a comprehensive set of rules for formatting PHP code.
This guide will walk you through implementing PSR-12 standards in your PHP projects using PHP-CS-Fixer, a powerful tool that automatically fixes your code to conform to these standards.
By the end of this guide, you'll have a fully automated setup that ensures all your PHP code follows PSR-12 standards, improving collaboration and code quality across your projects.
Why Use PSR-12?
Before diving into the technical details, let's understand why PSR-12 matters:
- Consistency: Standardized code formatting makes your codebase consistent and easier to read
- Collaboration: When everyone follows the same standards, collaboration becomes smoother
- Maintainability: Consistent code is easier to maintain and debug
- Industry Standard: PSR-12 is widely adopted in the PHP community and by major frameworks
- Modern PHP Support: PSR-12 includes rules for newer PHP features (7.x and 8.x)
PSR-12 extends PSR-2 and includes additional rules for newer PHP syntax features, making it the most up-to-date coding standard for modern PHP development.
Understanding PHP-CS-Fixer
PHP-CS-Fixer is a tool that analyzes and fixes your code to comply with coding standards. It's highly configurable and can be integrated into your development workflow in various ways.
Key features of PHP-CS-Fixer include:
- Support for PSR-1, PSR-2, PSR-12, and many other coding standards
- Ability to create custom rulesets or extend existing ones
- Integration with popular IDEs and code editors
- Command-line interface for manual or automated use
- CI/CD pipeline integration
PHP-CS-Fixer makes it easy to enforce coding standards without having to manually fix formatting issues.
Installation & Setup
There are several ways to install PHP-CS-Fixer. Here are the most common methods:
Composer (Recommended)
The recommended way to install PHP-CS-Fixer is through Composer:
# Install as a project dependency
composer require --dev friendsofphp/php-cs-fixer
# Or install globally
composer global require friendsofphp/php-cs-fixer
After installing globally, make sure the Composer bin directory is in your PATH to access the tool from anywhere.
PHAR File
You can also download the PHP-CS-Fixer PHAR (PHP Archive) file:
# Download the PHAR file
wget https://cs.symfony.com/download/php-cs-fixer-v3.phar -O php-cs-fixer
# Make it executable
chmod +x php-cs-fixer
# Move to a directory in your PATH
sudo mv php-cs-fixer /usr/local/bin/php-cs-fixer
To verify your installation, run:
php-cs-fixer --version
You should see output showing the version of PHP-CS-Fixer that you installed.
Basic Usage
Once installed, you can start using PHP-CS-Fixer to fix your code. Here are some basic commands:
# Fix a single file
php-cs-fixer fix path/to/file.php
# Fix all PHP files in a directory
php-cs-fixer fix path/to/directory
# Fix files but only show what would be changed without actually changing files
php-cs-fixer fix path/to/directory --dry-run
# Fix files and show detailed changes
php-cs-fixer fix path/to/directory --diff
By default, PHP-CS-Fixer uses a set of rules that may not exactly match PSR-12. To specifically use PSR-12 rules, you need to specify them:
php-cs-fixer fix path/to/directory --rules=@PSR12
However, for more control and consistency, it's better to create a configuration file.
Configuration Options
To customize PHP-CS-Fixer for your project, create a{" "}
.php-cs-fixer.php
or{" "}
.php-cs-fixer.dist.php
file in your project root:
in([
__DIR__ . '/src',
__DIR__ . '/tests',
])
->name('*.php')
->ignoreDotFiles(true)
->ignoreVCS(true);
$config = new PhpCsFixer\\Config();
return $config
->setRules([
'@PSR12' => true,
'array_syntax' => ['syntax' => 'short'],
'ordered_imports' => ['sort_algorithm' => 'alpha'],
'no_unused_imports' => true,
'trailing_comma_in_multiline' => true,
'phpdoc_scalar' => true,
'unary_operator_spaces' => true,
'binary_operator_spaces' => true,
'blank_line_before_statement' => [
'statements' => ['break', 'continue', 'declare', 'return', 'throw', 'try'],
],
'phpdoc_single_line_var_spacing' => true,
'phpdoc_var_without_name' => true,
'class_attributes_separation' => [
'elements' => [
'method' => 'one',
'property' => 'one',
],
],
'method_argument_space' => [
'on_multiline' => 'ensure_fully_multiline',
'keep_multiple_spaces_after_comma' => true,
],
'single_trait_insert_per_statement' => true,
])
->setFinder($finder)
->setUsingCache(true)
->setRiskyAllowed(true);
With this configuration file in place, you can simply run{" "}
php-cs-fixer fix
in your project root, and it will
apply all the specified rules.
PSR-12 Specific Rules
The @PSR12
ruleset includes all the rules required to
make your code PSR-12 compliant. Here are some key PSR-12 rules:
- Files MUST use only UTF-8 without BOM for PHP code
- Lines SHOULD be 80 characters or less
- There MUST be one blank line after namespace declarations
- Opening braces for classes MUST go on the next line
- Opening braces for methods MUST go on the next line
- Visibility MUST be declared on all properties and methods
- Control structure keywords MUST have one space after them
- Opening parentheses for control structures MUST NOT have a space after them
- Closing parentheses for control structures MUST NOT have a space before them
The full PSR-12 specification can be found on the{" "} PHP-FIG website .
Customizing Rules
While PSR-12 provides a solid foundation, you might want to add additional rules or customize existing ones. Here's how to extend the PSR-12 ruleset:
// In .php-cs-fixer.php
return $config
->setRules([
'@PSR12' => true,
// Additional rules
'array_syntax' => ['syntax' => 'short'],
'ordered_imports' => ['sort_algorithm' => 'alpha'],
'no_unused_imports' => true,
// Override PSR-12 rules if needed
'braces' => [
'allow_single_line_anonymous_class_with_empty_body' => true,
'allow_single_line_closure' => true,
],
])
->setFinder($finder);
You can find a complete list of available rules in the{" "} PHP-CS-Fixer documentation .
Command Line Options
PHP-CS-Fixer offers many command-line options to customize its behavior:
# Show all available rules
php-cs-fixer describe
# Show details about a specific rule
php-cs-fixer describe array_syntax
# Fix files using a specific config file
php-cs-fixer fix --config=.php-cs-fixer.custom.php
# Fix only specific files that match a pattern
php-cs-fixer fix --path-mode=intersection --path="src/Entity/*"
# Fix files but only show what would be changed
php-cs-fixer fix --dry-run --diff
# Fix files and show progress
php-cs-fixer fix --verbose
# Fix files using a specific PHP version
php-cs-fixer fix --using-cache=no --rules=@PHP80Migration
These options give you fine-grained control over how PHP-CS-Fixer processes your files.
IDE Integration
Integrating PHP-CS-Fixer with your IDE can significantly improve your workflow by automatically formatting code as you write or save.
Visual Studio Code
For VS Code, you can use the "PHP CS Fixer" extension:
- Install the "PHP CS Fixer" extension from the VS Code marketplace
- Configure the extension in your settings.json:
{
"php-cs-fixer.executablePath": "${"/path/to/extension"}/php-cs-fixer.phar",
"php-cs-fixer.onsave": true,
"php-cs-fixer.rules": "@PSR12",
"php-cs-fixer.config": ".php-cs-fixer.php",
"php-cs-fixer.allowRisky": false,
"editor.formatOnSave": true,
"[php]": {
"editor.defaultFormatter": "junstyle.php-cs-fixer"
}
}
With this configuration, your PHP files will be automatically formatted according to PSR-12 when you save them.
PhpStorm
PhpStorm has built-in support for PHP-CS-Fixer:
- Go to Settings/Preferences → Tools → External Tools
- Click the + button to add a new tool
- Configure the tool with the following settings:
- Name: PHP-CS-Fixer
- Program: Path to php-cs-fixer (e.g., /usr/local/bin/php-cs-fixer)
- Arguments: fix "$FileDir$/$FileName$" --config=$ProjectFileDir$/.php-cs-fixer.php
- Working directory: $ProjectFileDir$
You can also set up a File Watcher to automatically run PHP-CS-Fixer when you save a file:
- Go to Settings/Preferences → Tools → File Watchers
- Click the + button and select "Custom Template"
- Configure the watcher with similar settings to the External Tool
Sublime Text
For Sublime Text, you can use the "PHP CS Fixer" package:
- Install Package Control if you haven't already
- Install the "PHP CS Fixer" package
- Configure the package in your settings:
{
"php_cs_fixer_executable_path": "/usr/local/bin/php-cs-fixer",
"php_cs_fixer_additional_args": {"--rules": "@PSR12"},
"format_on_save": true,
"php_cs_fixer_on_save": true
}
CI Integration
Integrating PHP-CS-Fixer into your Continuous Integration (CI) pipeline ensures that all code contributions follow your formatting standards.
GitHub Actions
Here's an example GitHub Actions workflow that checks PHP code formatting:
# .github/workflows/php-cs-fixer.yml
name: PHP CS Fixer
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
php-cs-fixer:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Setup PHP
uses: shivammathur/setup-php@v2
with:
php-version: '8.1'
tools: php-cs-fixer
- name: Check code style
run: php-cs-fixer fix --dry-run --diff --config=.php-cs-fixer.php
This workflow will fail if any PHP files in your repository are not properly formatted according to your configuration.
GitLab CI
For GitLab CI, you can use a similar configuration:
# .gitlab-ci.yml
php-cs-fixer:
image: php:8.1-cli
stage: lint
before_script:
- apt-get update && apt-get install -y wget
- wget https://cs.symfony.com/download/php-cs-fixer-v3.phar -O php-cs-fixer
- chmod +x php-cs-fixer
- mv php-cs-fixer /usr/local/bin/php-cs-fixer
script:
- php-cs-fixer fix --dry-run --diff --config=.php-cs-fixer.php
only:
- merge_requests
- main
Troubleshooting Common Issues
Even with a well-configured formatter, you might encounter some issues. Here are solutions to common problems:
PHP-CS-Fixer Not Found
If you get a "command not found" error, make sure:
- PHP-CS-Fixer is installed correctly
- The executable is in your PATH (for global installations)
- You're using the correct path in your IDE configuration
Configuration File Not Found
If PHP-CS-Fixer can't find your configuration file:
- Make sure the file is named correctly (.php-cs-fixer.php or .php-cs-fixer.dist.php)
- Verify the file is in your project root or specify the path explicitly
- Check that the file has the correct PHP syntax
Conflicts with Other Tools
If you're using multiple formatting tools (e.g., PHP_CodeSniffer and PHP-CS-Fixer):
- Make sure they don't run simultaneously
- Consider using only one tool for formatting
- Ensure their configurations don't conflict
Handling Legacy Code
When applying PSR-12 to legacy codebases:
- Consider running PHP-CS-Fixer on a file-by-file basis
- Create a separate branch for formatting changes
- Review the changes carefully before merging
Best Practices
Follow these best practices to get the most out of PHP-CS-Fixer and PSR-12:
- Commit your configuration: Include your .php-cs-fixer.php file in version control
- Document your setup: Add instructions for setting up PHP-CS-Fixer in your project README
- Format before committing: Set up pre-commit hooks to format code automatically
- Enforce in CI: Reject pull requests with improperly formatted code
- Be consistent: Use the same configuration across all your projects
- Update regularly: Keep PHP-CS-Fixer updated to benefit from new features and fixes
- Start early: Implement formatting standards from the beginning of a project
For pre-commit hooks, you can use tools like Husky and lint-staged (for JavaScript/PHP projects) or Git's native pre-commit hooks.
Conclusion
Implementing PSR-12 standards with PHP-CS-Fixer is a valuable investment in your PHP projects. By automating code formatting, you eliminate style debates, improve code readability, and make collaboration smoother.
Remember that the goal of code formatting is not just aesthetic—it's about creating a consistent, maintainable codebase that's easier to work with for everyone involved.
With the setup described in this guide, you can ensure that all your PHP code follows PSR-12 standards automatically, letting you focus on what matters most: writing great PHP applications.