Python Code Styling with Black, isort & autopep8 | Web Formatter Blog
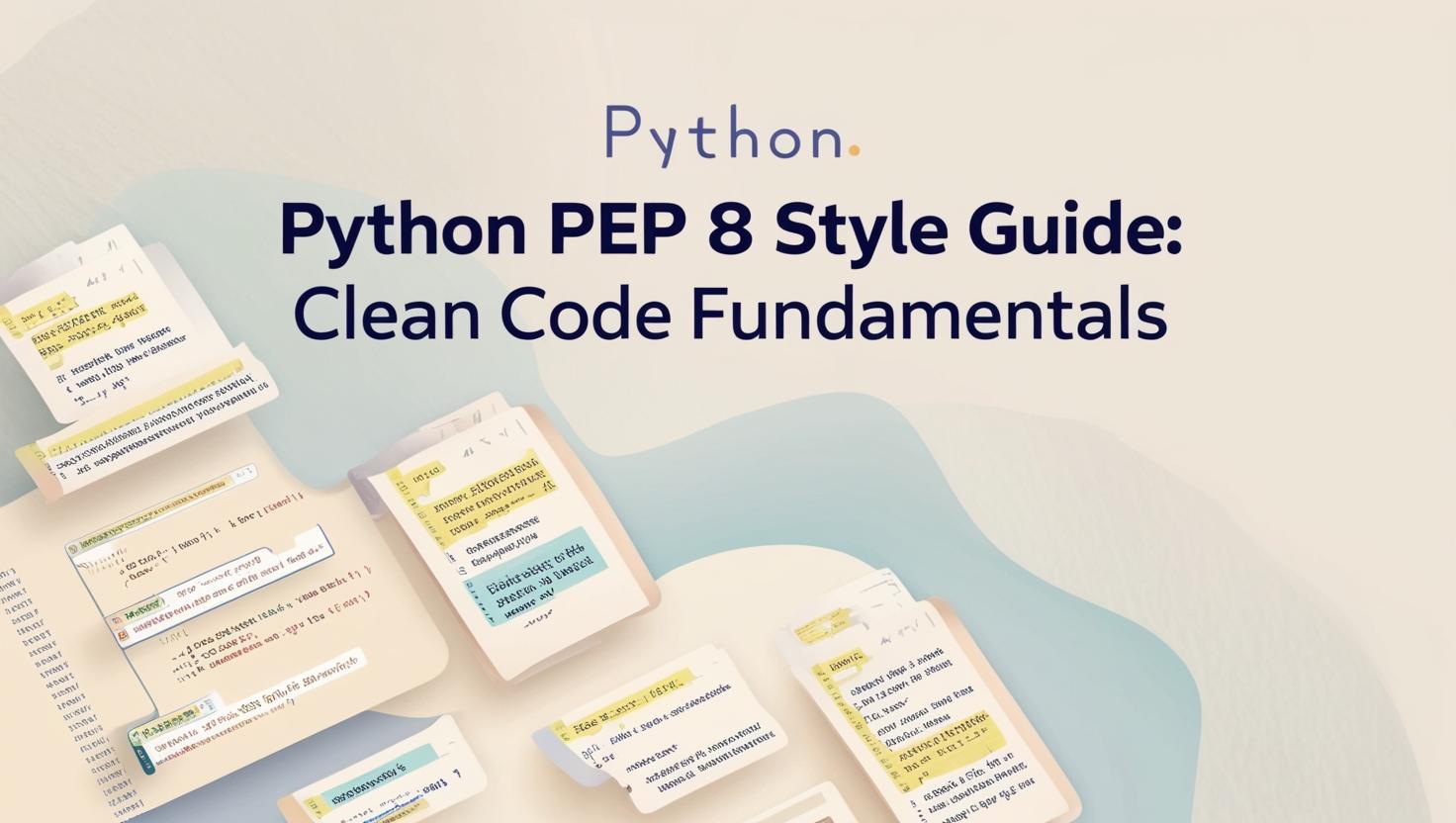
Introduction to Python Code Formatting
Consistent code formatting is essential for maintaining readability and reducing cognitive load when working in teams. For Python developers, several tools have emerged to automate formatting and ensure adherence to style guidelines. This article explores three of the most popular Python code formatters: Black, isort, and autopep8.
Whether you're a solo developer looking to standardize your own code or part of a large team trying to maintain consistency across a codebase, these tools can save time, reduce merge conflicts, and make code reviews focus on what matters—logic and functionality—rather than stylistic choices.
Understanding PEP 8
Before diving into specific tools, it's important to understand PEP 8, Python's official style guide. PEP 8 provides conventions for:
- Indentation (4 spaces per level)
- Maximum line length (79 characters for code, 72 for docstrings)
- Blank lines between functions and classes
- Import ordering and grouping
- Whitespace in expressions and statements
- Comments and docstrings
- Naming conventions
While PEP 8 is widely respected, manually ensuring compliance can be tedious. That's where automated formatters come in.
Black: The Uncompromising Code Formatter
Black bills itself as "the uncompromising Python code formatter." Its philosophy is simple: eliminate debates about code formatting by providing minimal configuration options. Black reformats entire files in place and is deterministic—the same input will always produce the same output.
Installing Black
Black can be installed using pip:
pip install black
For a specific version or to install in a virtual environment:
python -m pip install black==23.3.0
Using Black
- Format a single file: `black your_file.py`
- Format a directory: `black your_directory/`
- Check without writing: `black --check your_file.py`
Here's an example of how Black transforms code:
Black Configuration Options
While Black is intentionally limited in configuration, it does offer some options:
- Line length (default 88)
- Target Python versions
- String normalization
- Numeric literal handling
Settings can be specified in `pyproject.toml`:
[tool.black]
line-length = 88
target-version = ['py38']
include = '\\.pyi?$'
exclude = '''
/(
\\.git
| \\.hg
| \\.mypy_cache
| \\.tox
| \\.venv
| _build
| buck-out
| build
| dist
)/
'''
isort: Sorting Imports So You Don't Have To
While Black handles most formatting issues, it doesn't reorganize imports. That's where isort comes in—it automatically sorts, formats, and groups import statements according to PEP 8 guidelines.
Installing isort
pip install isort
Using isort
- Sort imports in a file: `isort your_file.py`
- Sort imports in a directory: `isort your_directory/`
- Check only: `isort --check-only your_file.py`
Here's how isort organizes imports:
Configuring isort
isort can be configured in `pyproject.toml`:
[tool.isort]
profile = "black"
line_length = 88
multi_line_output = 3
include_trailing_comma = true
force_grid_wrap = 0
use_parentheses = true
ensure_newline_before_comments = true
The `profile = "black"` setting ensures compatibility with Black.
autopep8: Automatically Format Code to PEP 8
autopep8 automatically formats Python code to conform to PEP 8. Unlike Black, autopep8 focuses on fixing specific PEP 8 violations and offers more granular control over which rules to enforce.
Installing autopep8
pip install autopep8
Using autopep8
- Format in place: `autopep8 --in-place your_file.py`
- Aggressive: `autopep8 --in-place --aggressive --aggressive your_file.py`
Here's how autopep8 transforms code:
Configuring autopep8
autopep8 can be configured via `setup.cfg`:
[pycodestyle]
max_line_length = 88
ignore = E203, W503
select = C,E,F,W,B,B950
exclude = .git,__pycache__,docs/,old/,build/,dist/
Integrating into Your Workflow
These formatting tools are most effective when integrated into your development workflow.
Using Pre-commit Hooks
Pre-commit hooks ensure code is formatted before it's committed. Example `.pre-commit-config.yaml`:
repos:
- repo: https://github.com/pycqa/isort
rev: 5.12.0
hooks:
- id: isort
- repo: https://github.com/psf/black
rev: 23.3.0
hooks:
- id: black
language_version: python3.10
- repo: https://github.com/pycqa/autopep8
rev: v2.0.2
hooks:
- id: autopep8
Install with `pre-commit install`.
CI/CD Integration
Integrate into GitHub Actions:
name: Code Quality
on: [push, pull_request]
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Python
uses: actions/setup-python@v4
with:
python-version: '3.10'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install black isort autopep8
- name: Check formatting with Black
run: black --check .
- name: Check imports with isort
run: isort --check-only --profile black .
- name: Check PEP 8 with autopep8
run: autopep8 --exit-code --recursive --diff .
IDE Integration
- VSCode: set `python.formatting.provider` to `black`, `editor.formatOnSave`: true, and organize imports on save.
- PyCharm: install Black plugin and configure External Tools for isort/autopep8.
- Jupyter: use `pip install black[jupyter]`.
Comparing the Tools
While all three tools improve code formatting, they serve slightly different purposes:
Best Practices
- Use them together: isort for imports, Black for overall formatting, and autopep8 for additional PEP 8 compliance.
- Consistent configuration across environments.
- Format entire codebase at once to avoid incremental diffs.
- Commit configuration files (`pyproject.toml`, etc.) to version control.
- Document formatting choices for new team members.
- Automate with pre-commit hooks and CI/CD.
Conclusion
Automated code formatting tools like Black, isort, and autopep8 free developers from the burden of manual formatting and style enforcement. By integrating these tools into your workflow, you can focus on writing functional, maintainable code while ensuring your codebase remains clean and consistent.
Consistent formatting not only improves readability but also reduces merge conflicts and keeps code reviews focused on logic rather than style. Start using these tools today to see immediate benefits in your Python projects.
Try Our Formatting Tools
Format your code with our free online tools:
Need more formatting tools?
Explore our complete collection of free online code formatting tools.
Browse All Formatters