CSS Formatter Reference: Stylelint & CSScomb Guide | Web Formatter Blog
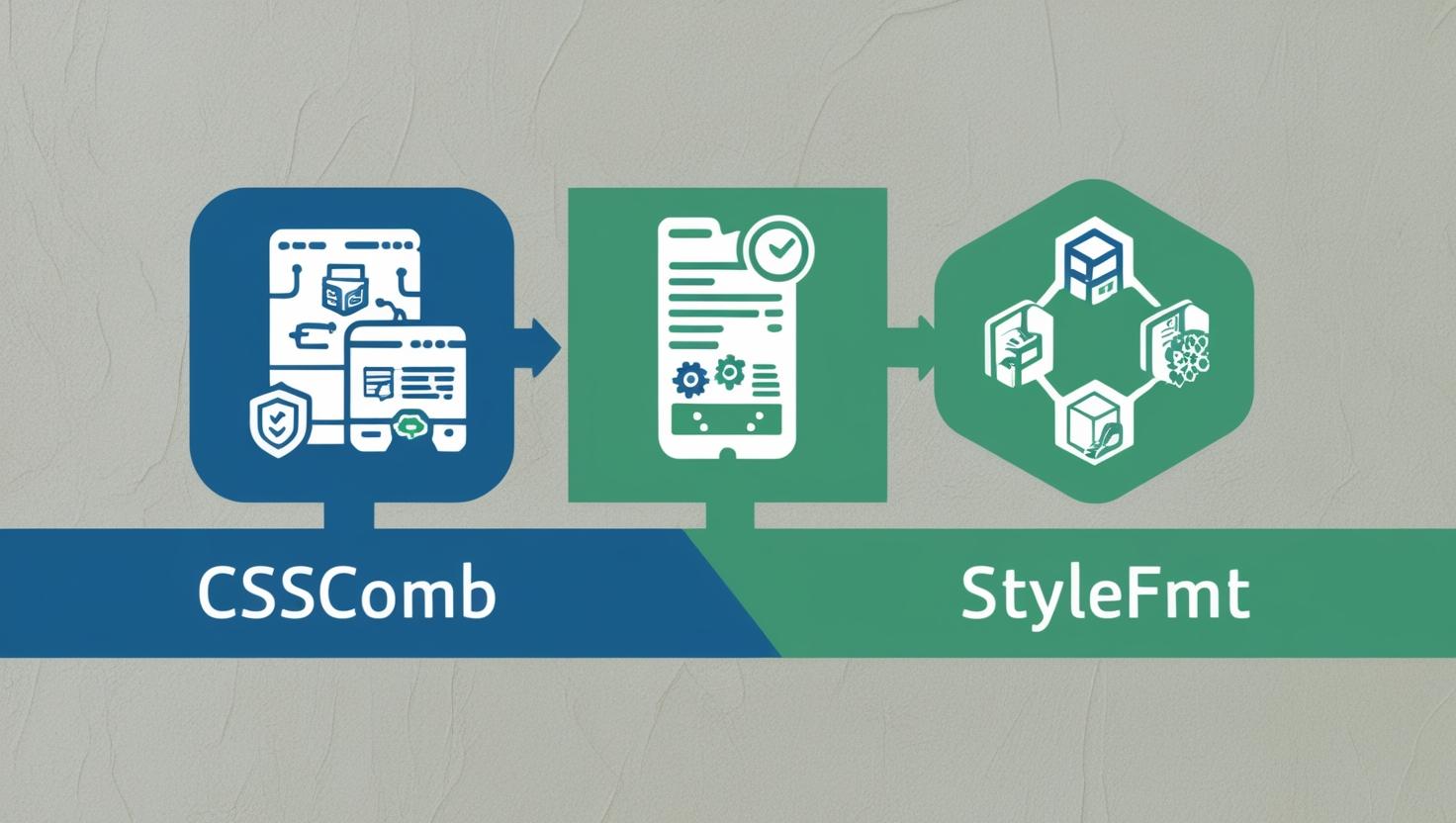
CSS Formatter Reference: Stylelint & CSScomb Guide
A comprehensive reference for maintaining clean, consistent CSS code with popular formatting tools.
Introduction to CSS Formatting
Consistent CSS formatting is crucial for maintainable codebases, especially on projects with multiple contributors. While CSS is flexible in its syntax, inconsistent styles, organization, and formatting can quickly lead to unmaintainable "CSS soup" that's difficult to debug and extend.
This guide explores two powerful tools in the CSS formatting ecosystem: Stylelint and CSScomb. These tools serve different but complementary roles in ensuring your stylesheets are clean, consistent, and follow best practices. Whether you're working on a small personal project or a large-scale application, these tools can significantly improve your CSS workflow.
Stylelint
Stylelint is a powerful, modern linter for CSS and CSS-like syntaxes. It helps catch errors, enforces consistent conventions, and prevents common mistakes. With over 170 built-in rules and a plugin ecosystem, Stylelint is highly customizable to fit your team's specific needs.
Installation & Setup
To install Stylelint using npm:
npm install --save-dev stylelint
For a standard configuration, you can use the recommended config:
npm install --save-dev stylelint-config-standard
Create a .stylelintrc.json
file in your project root:
{
"extends": "stylelint-config-standard",
"rules": {
"indentation": 2,
"color-hex-case": "lower",
"color-hex-length": "short"
}
}
To run Stylelint on your CSS files:
npx stylelint "**/*.css"
To automatically fix issues where possible:
npx stylelint "**/*.css" --fix
Configuration Options
Stylelint is highly configurable through its{" "}
.stylelintrc
file, which can be in JSON, YAML, or JS
format. The main configuration options include:
-
extends
: Predefined configurations to build upon -
plugins
: Additional rule sets from plugins -
rules
: Specific rule configurations -
ignoreFiles
: Glob patterns for files to ignore -
defaultSeverity
: Default rule violation severity
Example of a more comprehensive configuration:
{
"extends": [
"stylelint-config-standard",
"stylelint-config-recommended-scss"
],
"plugins": [
"stylelint-scss",
"stylelint-order"
],
"rules": {
"indentation": 2,
"string-quotes": "single",
"no-duplicate-selectors": true,
"color-hex-case": "lower",
"color-hex-length": "short",
"selector-no-qualifying-type": true,
"selector-combinator-space-after": "always",
"selector-attribute-quotes": "always",
"declaration-block-trailing-semicolon": "always",
"declaration-colon-space-before": "never",
"declaration-colon-space-after": "always",
"property-no-vendor-prefix": true,
"value-no-vendor-prefix": true,
"number-leading-zero": "always",
"function-url-quotes": "always",
"comment-whitespace-inside": "always",
"comment-empty-line-before": "always",
"selector-pseudo-element-colon-notation": "double",
"selector-pseudo-class-parentheses-space-inside": "never",
"media-feature-range-operator-space-before": "always",
"media-feature-range-operator-space-after": "always",
"media-feature-parentheses-space-inside": "never",
"media-feature-colon-space-before": "never",
"media-feature-colon-space-after": "always",
"order/properties-alphabetical-order": true
}
}
Essential Rules
Stylelint's rules are categorized into several groups:
Category | Examples | Purpose |
---|---|---|
Possible Errors |
color-no-invalid-hex ,{" "}
font-family-no-duplicate-names
|
Catch CSS syntax and usage errors |
Limit Language Features |
color-named ,{" "}
function-blacklist
|
Restrict usage of problematic language features |
Stylistic Issues |
indentation , string-quotes
|
Enforce consistent style and formatting |
Plugin Rules |
order/properties-alphabetical-order ,{" "}
scss/dollar-variable-pattern
|
Add specialized functionality via plugins |
Some particularly useful rules include:
-
no-duplicate-selectors
: Prevent duplicate selectors that can lead to confusion -
declaration-block-no-duplicate-properties
: Catch accidental property duplications -
shorthand-property-no-redundant-values
: Enforce concise shorthand properties -
selector-max-specificity
: Limit selector specificity to prevent specificity wars -
order/properties-alphabetical-order
: Organize properties for easier reading
Plugins & Extensions
Stylelint's functionality can be extended with plugins:
-
stylelint-scss
: SCSS-specific linting rules -
stylelint-order
: Property order enforcement -
stylelint-a11y
: Accessibility-focused linting rules -
stylelint-prettier
: Prettier integration for formatting -
stylelint-no-unsupported-browser-features
: Compatibility checking
Popular configuration presets include:
-
stylelint-config-standard
: Standard rules for CSS -
stylelint-config-recommended-scss
: SCSS-specific recommendations -
stylelint-config-prettier
: Disables rules that conflict with Prettier -
stylelint-config-idiomatic-order
: Logical property ordering
CSScomb
CSScomb is a coding style formatter specifically designed for CSS. It focuses on property ordering, indentation, and whitespace to ensure consistent formatting. Unlike Stylelint, which focuses on linting and catching errors, CSScomb's primary purpose is beautifying and organizing your CSS.
Installation & Setup
Install CSScomb using npm:
npm install --save-dev csscomb
Create a configuration file using one of the presets:
npx csscomb --create-configuration csscomb.json
Run CSScomb on your CSS files:
npx csscomb path/to/styles
Configuration Options
CSScomb's configuration includes options for:
- Property ordering
- Indentation style and size
- Whitespace handling
- Line breaks and blank lines
- Color format preferences
- Quote style for strings
Example CSScomb configuration:
{
"remove-empty-rulesets": true,
"always-semicolon": true,
"color-case": "lower",
"block-indent": " ",
"color-shorthand": true,
"element-case": "lower",
"eof-newline": true,
"leading-zero": true,
"quotes": "single",
"space-before-colon": "",
"space-after-colon": " ",
"space-before-combinator": " ",
"space-after-combinator": " ",
"space-between-declarations": "\\n",
"space-before-opening-brace": " ",
"space-after-opening-brace": "\\n",
"space-after-selector-delimiter": "\\n",
"space-before-selector-delimiter": "",
"space-before-closing-brace": "\\n",
"strip-spaces": true,
"sort-order": [
[
"position",
"top",
"right",
"bottom",
"left",
"z-index",
"display",
"flex",
"flex-direction",
"flex-flow",
"flex-wrap"
],
[
"width",
"height",
"margin",
"padding",
"border",
"background",
"color",
"font",
"text-align"
],
[
"content",
"cursor"
]
]
}
Property Sorting
One of CSScomb's most valuable features is its ability to sort CSS properties in a consistent order. There are several common approaches to property ordering:
- Alphabetical: Easy to maintain but doesn't group related properties
- Type-based: Groups properties by type (positioning, display, box model, typography, etc.)
- Concentric: Orders properties from outside in (margin, border, padding, content)
- Related: Groups related properties together (e.g., all flex properties)
Here's an example of type-based ordering, which many developers prefer for its logical grouping:
Presets & Templates
CSScomb comes with several built-in presets that you can use as a starting point:
-
csscomb
: Default CSScomb style -
zen
: Zen coding style -
yandex
: Yandex's coding style
To use a preset:
npx csscomb -p zen path/to/styles
You can also create custom presets by modifying an existing one or starting from scratch, then save it to share across projects or with your team.
Comparing Stylelint & CSScomb
Feature Comparison
While Stylelint and CSScomb may seem to overlap, they serve different primary purposes:
Feature | Stylelint | CSScomb |
---|---|---|
Primary Focus | Linting & error detection | Formatting & property ordering |
Rules/Checks | 170+ built-in rules | ~30 formatting options |
Preprocessor Support | SCSS, Less, PostCSS | SCSS, Less |
Auto-fix | Yes, for some rules | Yes, full reformatting |
Plugin System | Extensive | Limited |
Active Development | Very active | Less active |
Community Size | Large | Moderate |
Best Use Cases
The tools work best in different scenarios:
-
Stylelint shines when:
- You need comprehensive linting to catch errors
- You're working with a large team that needs clear style guidelines
- You use modern CSS features and need validation
- You work with CSS preprocessors extensively
-
CSScomb excels when:
- Property ordering is a priority
- You need to clean up and standardize legacy CSS
- You want simple, focused formatting without extensive validation
- You prefer configuration simplicity over extensibility
Many teams use both tools together: Stylelint for comprehensive linting and error prevention, and CSScomb for property ordering and specific formatting preferences.
Integration with Development Workflows
Code Editor Integration
Both tools integrate with popular code editors:
-
Visual Studio Code:
- Stylelint:{" "} Stylelint extension
- CSScomb:{" "} CSScomb extension
-
Sublime Text:
- Stylelint:{" "} SublimeLinter-stylelint
- CSScomb:{" "} CSScomb package
- WebStorm/PhpStorm: Both have built-in support for Stylelint and CSScomb
-
Vim:
- Stylelint:{" "} ALE {" "} with Stylelint integration
- CSScomb:{" "} vim-csscomb
For VS Code, you can configure format-on-save with Stylelint:
{
"editor.codeActionsOnSave": {
"source.fixAll.stylelint": true
},
"stylelint.validate": ["css", "scss"]
}
CI/CD Pipeline Integration
Incorporating these tools into your CI/CD pipeline ensures that all code meets your style standards before being merged or deployed:
# Example GitHub Actions workflow
name: CSS Linting
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: 16
- name: Install dependencies
run: npm ci
- name: Run Stylelint
run: npx stylelint "**/*.css" "**/*.scss"
- name: Run CSScomb check
run: npx csscomb -l "**/*.css" "**/*.scss"
The -l
flag with CSScomb lists files that would be
modified without making changes, which is ideal for CI checks.
For pre-commit hooks, you can use tools like Husky and lint-staged:
// package.json
{
"husky": {
"hooks": {
"pre-commit": "lint-staged"
}
},
"lint-staged": {
"*.{css,scss}": [
"stylelint --fix",
"csscomb"
]
}
}
Working with Preprocessors
SASS and LESS Support
Both tools support SASS and LESS preprocessors, but require additional configuration:
For Stylelint with SCSS:
npm install --save-dev stylelint-scss stylelint-config-recommended-scss
{
"extends": "stylelint-config-recommended-scss",
"plugins": [
"stylelint-scss"
],
"rules": {
"scss/dollar-variable-pattern": "^[a-z][a-zA-Z0-9]+$",
"scss/selector-no-redundant-nesting-selector": true
}
}
For CSScomb with preprocessors, include the file extensions in your command:
npx csscomb "**/*.scss" "**/*.less"
PostCSS Integration
Both tools can be integrated with PostCSS:
Stylelint as a PostCSS plugin:
const postcss = require('postcss');
const stylelint = require('stylelint');
postcss([
stylelint({ /* options */ }),
])
.process(css, { from: 'src/style.css', to: 'dist/style.css' })
.then(result => {
console.log('CSS linted!');
});
CSScomb as a PostCSS plugin requires postcss-csscomb:
npm install --save-dev postcss postcss-csscomb
const postcss = require('postcss');
const csscomb = require('postcss-csscomb');
postcss([
csscomb({ config: './csscomb.json' })
])
.process(css, { from: 'src/style.css', to: 'dist/style.css' })
.then(result => {
console.log('CSS formatted!');
});
CSS Formatting Best Practices
File Organization
Beyond individual file formatting, consider these organization best practices:
- Separate concerns: Split CSS into logical files by component or function
- Use imports strategically: Bundle common styles, import specific ones conditionally
- Follow a naming system: Consider methodologies like BEM, SMACSS, or OOCSS
- Organize with comments: Use section comments to delineate major parts of files
- Consider CSS modules: For component-based architectures, use CSS modules or scoped styles
Naming Conventions
Consistent naming significantly improves CSS maintainability:
-
BEM (Block, Element, Modifier):{" "}
.block__element--modifier
-
Use meaningful names: Prefer descriptive names
over presentation (
.primary-button
vs{" "}.red-button
) - Avoid excessive nesting: Keep selector specificity manageable
- Be consistent with case: Choose kebab-case, camelCase, or another style and stick with it
Here's an example of BEM naming:
.card {
background: white;
border-radius: 4px;
}
.card__title {
font-size: 18px;
font-weight: bold;
}
.card__content {
padding: 16px;
}
.card--featured {
border: 2px solid gold;
}
.card--disabled {
opacity: 0.5;
}
Formatting Guidelines
Some universally accepted formatting guidelines include:
- One selector per line in multi-selector rulesets
-
Space after property colon (
color: #000
notcolor:#000
) - End all declarations with a semicolon, even the last one for consistency
-
Use shorthand properties where applicable (
margin: 10px 20px
vs individual margins) - Group related properties for better readability
- Use consistent quotes (single or double) throughout the codebase
- Use lowercase selectors and property values for consistency
- Break long selector lists after commas
Conclusion
Consistent CSS formatting is an investment that pays dividends throughout a project's lifecycle. By using tools like Stylelint and CSScomb, you can automatically enforce coding standards and best practices, freeing your team to focus on creating great styles rather than debating formatting preferences.
For optimal results, consider:
- Using Stylelint for comprehensive linting and error prevention
- Adding CSScomb for consistent property ordering and additional formatting
- Integrating both into your editor and CI pipeline
- Creating team-specific configurations that reflect your project's needs
- Documenting your CSS guidelines beyond what the tools enforce
With these tools and practices in place, your CSS codebase will remain clean, consistent, and maintainable, even as it grows and evolves with your project.