SvelteKit & Prettier: Styling .svelte Components | Web Formatter Blog
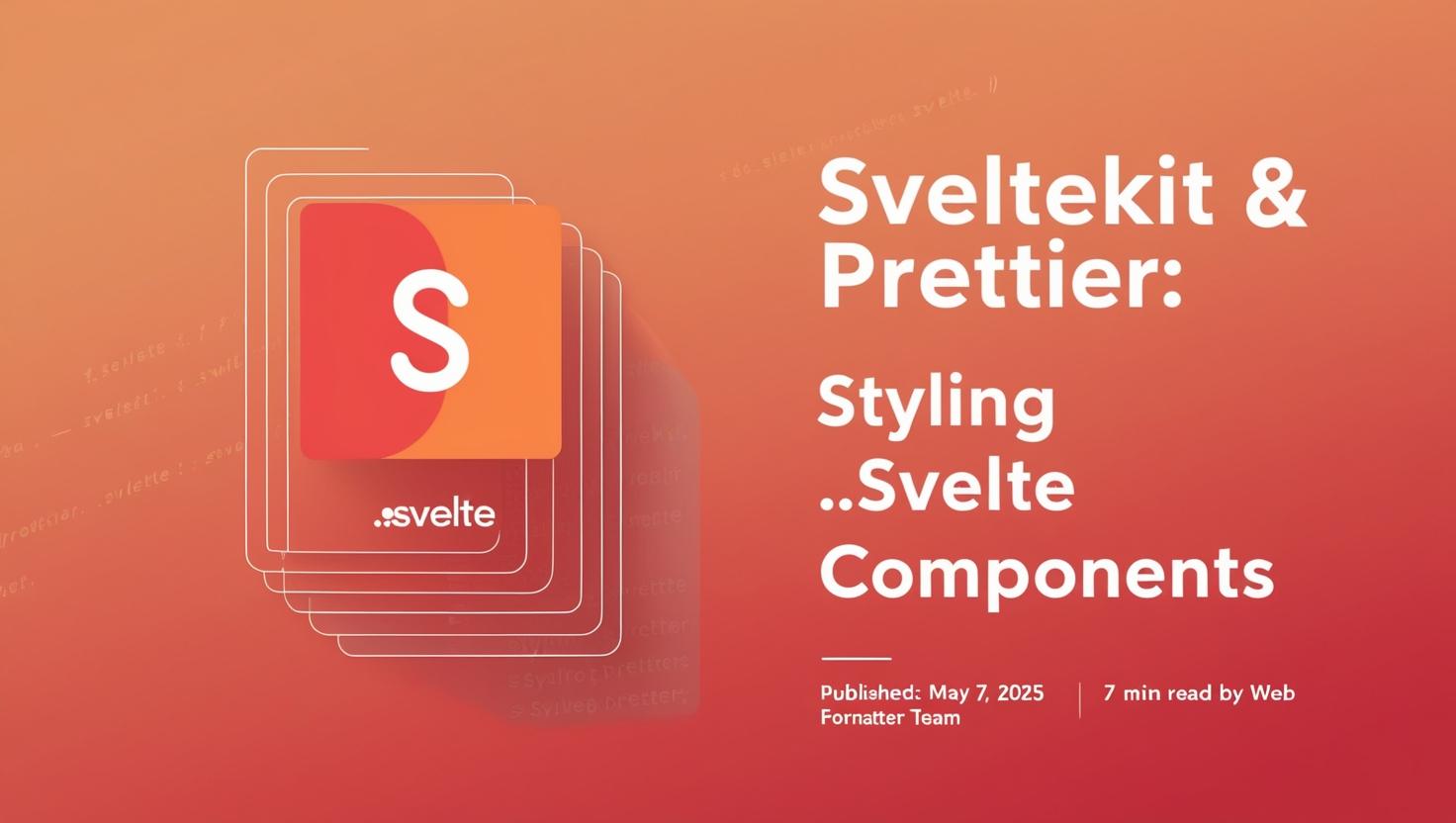
SvelteKit & Prettier: Styling .svelte Components
A comprehensive guide to formatting Svelte components in SvelteKit projects using Prettier.
Introduction to Svelte Formatting
Svelte has gained significant popularity as a component-based
framework that compiles at build time, resulting in highly
optimized vanilla JavaScript. With SvelteKit, developers can build
complete web applications using Svelte's component model. However,
maintaining consistent code style across
.svelte
files presents unique challenges due to their
mixed content nature.
Svelte components combine HTML, CSS, and JavaScript in a single file, making traditional formatters insufficient without proper configuration. This guide focuses on using Prettier, a popular code formatter, to maintain consistent styling in Svelte components within SvelteKit projects.
Why Format Svelte Components?
Consistent code formatting offers several benefits for Svelte development:
- Improved readability: Consistent formatting makes code easier to scan and understand
- Reduced cognitive load: Developers don't need to make style decisions while coding
- Easier collaboration: Consistent style reduces friction in team environments
- Cleaner diffs: Version control changes focus on content rather than formatting
- Faster onboarding: New team members can quickly adapt to the codebase's style
For Svelte specifically, proper formatting helps maintain clarity between the three main sections of a component: script, markup, and style.
Setting Up Prettier for SvelteKit
Prettier is an opinionated code formatter that supports many languages, including JavaScript, HTML, and CSS. To properly format Svelte components, you'll need Prettier and a Svelte-specific plugin.
Installation
First, install Prettier and the Svelte plugin as development dependencies:
# Using npm
npm install --save-dev prettier prettier-plugin-svelte
# Using yarn
yarn add --dev prettier prettier-plugin-svelte
# Using pnpm
pnpm add --save-dev prettier prettier-plugin-svelte
Prettier will automatically detect and use the Svelte plugin when
formatting .svelte
files.
Configuration
Create a .prettierrc
or{" "}
prettier.config.js
file in your project root to
configure Prettier:
{
"semi": true,
"tabWidth": 2,
"printWidth": 100,
"singleQuote": true,
"trailingComma": "es5",
"plugins": ["prettier-plugin-svelte"],
"pluginSearchDirs": ["."],
"overrides": [{ "files": "*.svelte", "options": { "parser": "svelte" } }]
}
You can also create a .prettierignore
file to exclude
certain files or directories from formatting:
node_modules
.svelte-kit
build
package
.env
.env.*
!.env.example
The Svelte Prettier Plugin
The prettier-plugin-svelte
package extends Prettier
to understand Svelte's syntax and formatting needs. It handles the
unique aspects of Svelte components, including:
- Script and style tags with their respective languages
- Svelte-specific syntax like directives and shorthand attributes
- Component markup with HTML-like syntax and Svelte extensions
- Proper indentation across all sections of a component
Plugin Options
The Svelte plugin provides several Svelte-specific formatting options you can add to your Prettier configuration:
{
"svelteSortOrder": "options-scripts-markup-styles",
"svelteStrictMode": false,
"svelteBracketNewLine": true,
"svelteAllowShorthand": true,
"svelteIndentScriptAndStyle": true
}
Let's explore these options in detail:
- svelteSortOrder: Controls the order of component sections (options, scripts, markup, styles)
- svelteStrictMode: When true, enforces HTML void elements to have closing tags
- svelteBracketNewLine: Controls whether to put the closing angle bracket on a new line in multiline elements
-
svelteAllowShorthand: Enables or disables
shorthand attribute syntax (
{"{"}
vs{" "}prop={"{prop}"}
) - svelteIndentScriptAndStyle: Controls whether to indent code inside script and style tags
Formatting Examples
Let's look at how Prettier formats different parts of a Svelte component.
Script Block Formatting
The script block contains JavaScript or TypeScript code and follows standard Prettier JavaScript formatting rules:
Template Formatting
The template section contains HTML-like markup with Svelte directives and expressions:
Style Block Formatting
The style block contains CSS or preprocessor code and follows Prettier's CSS formatting rules:
IDE Integration
Integrating Prettier with your IDE enables automatic formatting on save or with keyboard shortcuts.
Visual Studio Code
For VS Code, install the Prettier extension and configure it for Svelte:
- Install the "Prettier - Code formatter" extension
- Install the "Svelte for VS Code" extension
- Configure VS Code settings (settings.json):
{
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true,
"[svelte]": {
"editor.defaultFormatter": "svelte.svelte-vscode"
},
"prettier.documentSelectors": ["**/*.svelte"]
}
WebStorm / IntelliJ
For JetBrains IDEs:
- Install the "Svelte" plugin
- Go to Settings → Languages & Frameworks → JavaScript → Prettier
- Enable "Automatic Prettier configuration" and "Run on save"
-
Add
.svelte
to the list of file types if not already included
Vim / Neovim
For Vim or Neovim, you can use plugins like "vim-prettier" or "coc-prettier":
" Using vim-plug with vim-prettier
Plug 'prettier/vim-prettier', { 'do': 'yarn install --frozen-lockfile --production' }
" Configuration
let g:prettier#autoformat = 1
let g:prettier#autoformat_require_pragma = 0
let g:prettier#exec_cmd_path = "~/.nvm/versions/node/v16.14.0/bin/prettier"
" Add .svelte to the list of file types
let g:prettier#config#parser = 'babylon'
autocmd BufWritePre *.svelte PrettierAsync
CI Integration
Enforcing consistent formatting in your continuous integration pipeline ensures all code meets your project's standards.
GitHub Actions
Here's an example GitHub Actions workflow that checks Svelte formatting:
# .github/workflows/prettier.yml
name: Prettier
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
prettier:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: '16'
cache: 'npm'
- name: Install dependencies
run: npm ci
- name: Check formatting
run: npx prettier --check "**/*.{js,ts,svelte,css,scss,json,md}"
GitLab CI
For GitLab CI, you can use a similar configuration:
# .gitlab-ci.yml
prettier:
image: node:16
stage: lint
script:
- npm ci
- npx prettier --check "**/*.{js,ts,svelte,css,scss,json,md}"
only:
- merge_requests
- main
Troubleshooting Common Issues
Here are solutions to common issues when formatting Svelte components:
-
Plugin not detected: If Prettier doesn't detect
the Svelte plugin, try adding
"pluginSearchDirs": ["."]
to your Prettier config. -
Formatting conflicts with ESLint: Install{" "}
eslint-config-prettier
to disable ESLint rules that might conflict with Prettier. - Inconsistent formatting in a team: Ensure everyone uses the same Prettier and plugin versions by specifying exact versions in package.json.
-
Formatting breaking Svelte syntax: Make sure
you're using the latest version of
prettier-plugin-svelte
that supports all Svelte syntax features.
If you encounter issues with specific Svelte syntax, you can temporarily disable Prettier for sections of code:
Content
Best Practices
Follow these best practices for formatting Svelte components:
-
Commit your Prettier configuration: Include{" "}
.prettierrc
and.prettierignore
in version control - Use a pre-commit hook: Set up husky and lint-staged to format code before committing
-
Format the entire codebase: Run{" "}
npx prettier --write .
after setting up Prettier to format all files - Document your formatting choices: Add a section in your README about code style and formatting
- Keep plugins updated: Regularly update Prettier and the Svelte plugin to get the latest improvements
- Be consistent with quotes and semicolons: Choose a style and stick with it across your project
- Use reasonable line length: 80-100 characters is typically a good balance for readability
For SvelteKit projects specifically, consider adding a script to your package.json:
{
"scripts": {
"format": "prettier --write .",
"format:check": "prettier --check ."
}
}
Conclusion
Proper formatting of Svelte components is essential for maintaining a clean, readable codebase in SvelteKit projects. With Prettier and the Svelte plugin, you can automate formatting and ensure consistency across your entire project.
By following the setup instructions and best practices outlined in this guide, you'll eliminate formatting debates and allow your team to focus on building great Svelte applications rather than worrying about code style.
Remember that while formatting is important, it's just one aspect of code quality. Combine it with other tools like ESLint for static analysis and testing frameworks to ensure your SvelteKit project is not just well-formatted, but also well-structured and reliable.