Symfony Code Styling with EasyCodingStandard | Web Formatter Blog
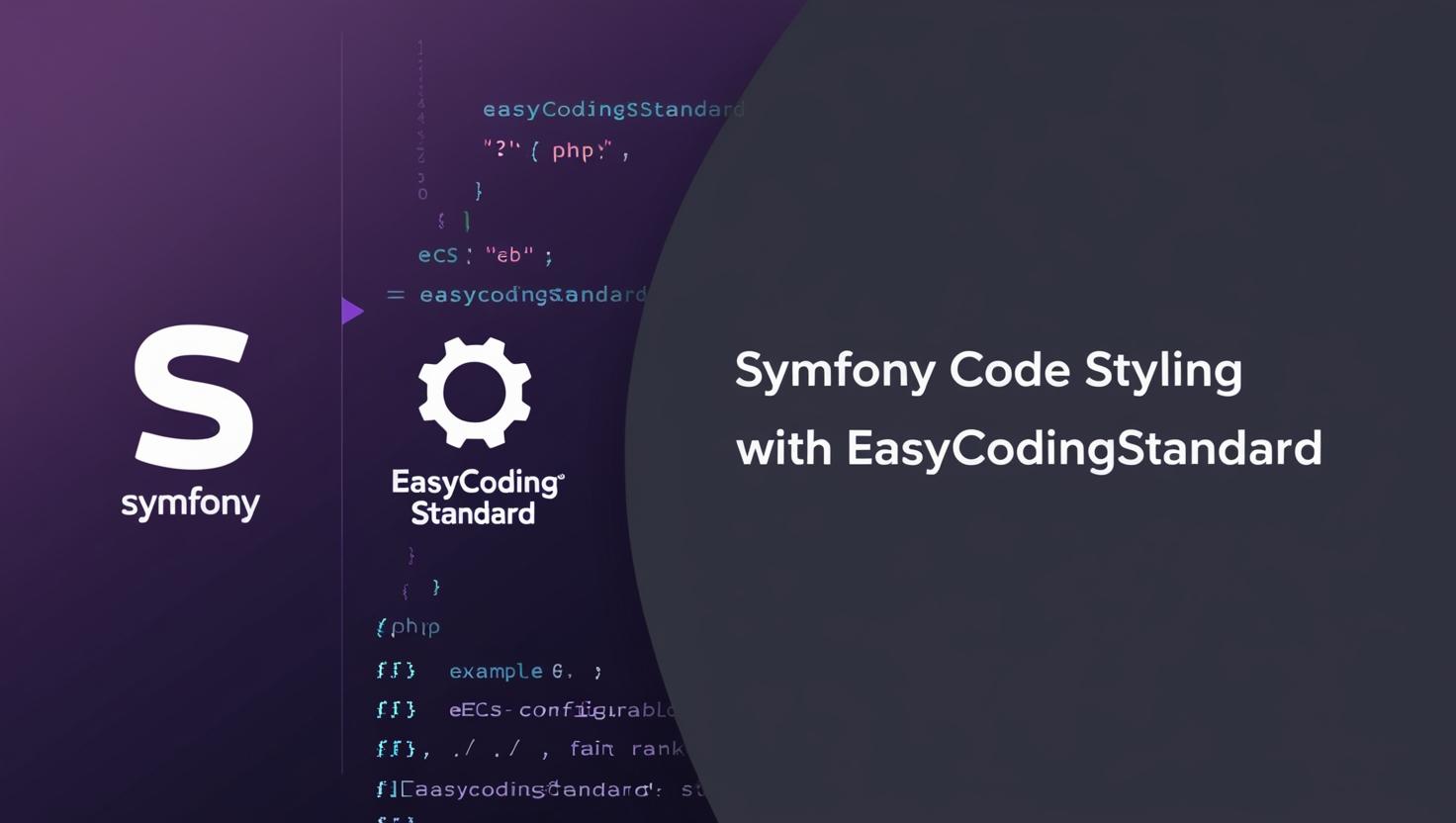
Symfony Code Styling with EasyCodingStandard
A comprehensive guide to formatting Symfony code for improved readability and maintainability.
Introduction to Symfony Code Styling
Symfony is one of the most popular PHP frameworks, known for its robust architecture and flexibility. As with any large-scale project, maintaining consistent code style across your Symfony applications is crucial for readability, maintainability, and collaboration.
In this comprehensive guide, we'll explore how to leverage EasyCodingStandard (ECS) to maintain beautiful, consistent PHP code across your Symfony projects, ensuring both readability and adherence to community best practices.
Why Format Your Symfony Code?
Consistent code formatting in Symfony projects offers numerous benefits:
- Improved readability: Consistently formatted code is easier to scan and comprehend
- Reduced cognitive load: Developers can focus on logic rather than style discrepancies
- Easier onboarding: New team members can quickly adapt to your codebase's style
- Faster code reviews: Reviewers can focus on substance rather than style
- Bug prevention: Many formatting rules catch potential issues before they become bugs
- Enforced best practices: Tools like ECS encode Symfony and PHP community standards
The Symfony community has established clear coding standards, documented in the Symfony Coding Standards . EasyCodingStandard makes it simple to apply these standards to your projects.
Understanding EasyCodingStandard
EasyCodingStandard (ECS) is a powerful tool that combines the capabilities of PHP-CS-Fixer and PHP_CodeSniffer into a single, unified interface. It helps identify and fix:
- Style violations: Inconsistent whitespace, braces, indentation, etc.
- Code structure issues: Class and method organization, naming conventions, etc.
- Documentation problems: Missing or malformed docblocks, parameter descriptions, etc.
- Symfony-specific standards: Adherence to Symfony's coding conventions
ECS simplifies the process of maintaining code quality by providing:
- A unified configuration system for both PHP-CS-Fixer and PHP_CodeSniffer
- Predefined rule sets for common standards (PSR-12, Symfony, etc.)
- The ability to customize and extend rules to match your project's needs
- Parallel processing for faster analysis of large codebases
- Automatic fixing of many common issues
Installation
Installing EasyCodingStandard
The recommended way to install ECS is via Composer:
composer require --dev symplify/easy-coding-standard
For a Symfony project, you'll typically want to install it as a development dependency:
composer require --dev symplify/easy-coding-standard
Basic Usage
Using EasyCodingStandard
To analyze all PHP files in your project with ECS:
vendor/bin/ecs check src
To analyze specific files or directories:
vendor/bin/ecs check src/Controller src/Entity/User.php
The output will show violations with their locations and explanations:
[ERROR] src/Controller/UserController.php
------------------------------------------------------------------------------------------------------
• Line 15: There must be exactly 1 space between the method name and opening parenthesis.
---------- begin diff ----------
- public function index ()
+ public function index()
----------- end diff -----------
[ERROR] src/Entity/User.php
------------------------------------------------------------------------------------------------------
• Line 25: Property \$createdAt type is missing a native type hint.
---------- begin diff ----------
- private $createdAt;
+ private \DateTime $createdAt;
----------- end diff -----------
[ERROR] Found 2 errors that need to be fixed.
Auto-correction with ECS
ECS can automatically fix many issues with the --fix
{" "}
flag:
vendor/bin/ecs check src --fix
To see what would be fixed without actually changing files:
vendor/bin/ecs check src --fix --dry-run
For faster processing on large codebases, use parallel processing:
vendor/bin/ecs check src --fix --parallel
Configuration
ecs.php Configuration
ECS is configured through an ecs.php
file in your
project's root directory:
parameters();
// Paths to check
$parameters->set(Option::PATHS, [
__DIR__ . '/src',
__DIR__ . '/tests',
]);
// Skip some files or patterns
$parameters->set(Option::SKIP, [
__DIR__ . '/src/Kernel.php',
__DIR__ . '/src/bootstrap.php',
]);
// Use predefined sets
$containerConfigurator->import(SetList::PSR_12);
$containerConfigurator->import(SetList::SYMFONY);
// Configure specific rules
$services = $containerConfigurator->services();
$services->set(ArraySyntaxFixer::class)
->call('configure', [[
'syntax' => 'short',
]]);
};
This example configuration:
-
Checks files in the
src
andtests
{" "} directories - Skips specific files that shouldn't be checked
- Imports the PSR-12 and Symfony rule sets
- Configures the ArraySyntaxFixer to enforce short array syntax
Using Predefined Sets
ECS comes with several predefined rule sets that you can import:
// Import multiple sets
$containerConfigurator->import(SetList::PSR_12);
$containerConfigurator->import(SetList::SYMFONY);
$containerConfigurator->import(SetList::CLEAN_CODE);
$containerConfigurator->import(SetList::DOCTRINE_ANNOTATIONS);
Common sets include:
-
SetList::PSR_12
: PSR-12 coding standard -
SetList::SYMFONY
: Symfony coding standard -
SetList::CLEAN_CODE
: Rules for cleaner code -
SetList::COMMON
: Common PHP rules -
SetList::DOCTRINE_ANNOTATIONS
: Rules for Doctrine annotations -
SetList::ARRAY
: Rules for array formatting
Customizing Rules
You can customize individual rules in your configuration:
use PhpCsFixer\\Fixer\\Whitespace\\LineEndingFixer;
use PhpCsFixer\\Fixer\\ClassNotation\\VisibilityRequiredFixer;
// Configure specific rules
$services = $containerConfigurator->services();
// Set line endings to LF
$services->set(LineEndingFixer::class);
// Require visibility declarations for properties and methods
$services->set(VisibilityRequiredFixer::class)
->call('configure', [[
'elements' => ['property', 'method', 'const'],
]]);
You can also disable specific rules from sets:
// Skip specific rules
$parameters->set(Option::SKIP, [
// Skip a specific rule
PhpCsFixer\\Fixer\\ClassNotation\\FinalClassFixer::class => null,
// Skip a rule for specific files
PhpCsFixer\\Fixer\\Strict\\DeclareStrictTypesFixer::class => [
__DIR__ . '/src/Legacy',
],
]);
Symfony-Specific Configuration
For Symfony projects, you'll want to use the Symfony rule set and potentially add some Symfony-specific configurations:
parameters();
// Paths to check (Symfony structure)
$parameters->set(Option::PATHS, [
__DIR__ . '/src',
__DIR__ . '/tests',
__DIR__ . '/config',
]);
// Skip some Symfony-specific files
$parameters->set(Option::SKIP, [
__DIR__ . '/src/Kernel.php',
__DIR__ . '/config/bundles.php',
__DIR__ . '/public/index.php',
]);
// Use Symfony standards
$containerConfigurator->import(SetList::SYMFONY);
// Add additional rules for Symfony projects
$services = $containerConfigurator->services();
// Require strict types declaration
$services->set(DeclareStrictTypesFixer::class);
// Configure Yoda style (Symfony prefers Yoda conditions)
$services->set(YodaStyleFixer::class)
->call('configure', [[
'equal' => true,
'identical' => true,
'less_and_greater' => false,
]]);
// Order PHPDoc types
$services->set(PhpdocTypesOrderFixer::class)
->call('configure', [[
'null_adjustment' => 'always_last',
'sort_algorithm' => 'alpha',
]]);
};
Code Examples
PHP Formatting Examples
ECS with Symfony rules applies several improvements:
-
Adds
declare(strict_types=1);
statement - Converts PHPDoc route annotations to PHP 8 attributes
- Adds return type declarations
- Adds parameter type hints
- Fixes spacing around method names and parentheses
- Standardizes array syntax to short array notation
- Fixes array key spacing
- Converts boolean constants to lowercase
-
Applies Yoda conditions (e.g.,
1 === $id
instead of{" "}$id == 1
) -
Standardizes array syntax in
render()
calls - Adds trailing commas in multi-line arrays
Twig Template Formatting
While ECS primarily focuses on PHP files, you can use additional tools like Twig-CS-Fixer for Twig templates. Here's an example of formatting Twig templates:
Twig formatting improvements include:
- Consistent spacing inside Twig tags and variables
- Proper indentation of nested HTML and Twig structures
- Consistent spacing in control structures
- Proper alignment of opening and closing tags
Symfony-Specific Formatting
Symfony-specific formatting improvements include:
- Converting Doctrine annotations to PHP 8 attributes
- Adding property type declarations
- Adding return type declarations
- Adding parameter type hints
-
Using
self
as return type for fluent interfaces -
Properly importing classes (e.g.,
DateTime
) - Adding nullable type hints where appropriate
- Initializing nullable properties
- Adding blank lines between methods for better readability
Editor Integration
Integrating ECS with your editor allows for real-time feedback and automatic formatting.